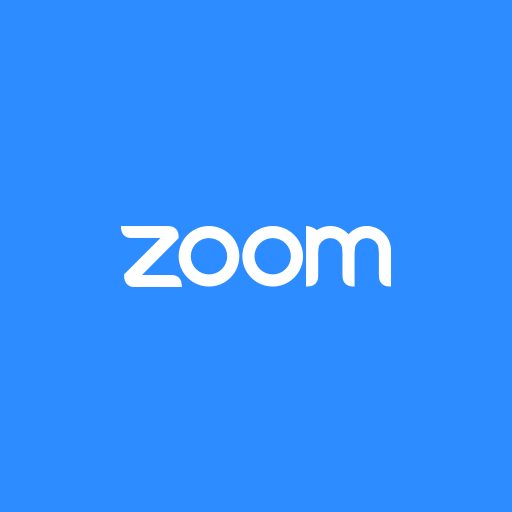
Zoom Video Call
VideoHow to Access Zoom Public API Docs
If you're interested in using the Zoom public API, you'll need to take a look at the API reference documentation. This documentation is available on the Zoom Marketplace website, and it provides detailed information on the available endpoints.
To make things easier for developers, the Zoom API is RESTful, which means that it follows a standard set of conventions for HTTP requests and responses. This means that you can use any programming language to access the Zoom API, including JavaScript.
Zoom API Example Code in JavaScript
Here are some examples of how you can use the Zoom API with JavaScript:
Create a Zoom Meeting
fetch(
'https://api.zoom.us/v2/users/{userId}/meetings',
{
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer {accessToken}',
},
body: JSON.stringify({
topic: 'Example Meeting',
type: 1,
start_time: '2021-01-01T00:00:00Z',
duration: 60,
timezone: 'America/New_York',
agenda: 'This is an example meeting created via Zoom API.',
}),
}
)
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
Get a List of Zoom Meetings
fetch(
'https://api.zoom.us/v2/users/{userId}/meetings',
{
headers: {
'Authorization': 'Bearer {accessToken}',
},
}
)
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
Update a Zoom Meeting
fetch(
'https://api.zoom.us/v2/meetings/{meetingId}',
{
method: 'PATCH',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer {accessToken}',
},
body: JSON.stringify({
topic: 'Updated Example Meeting',
agenda: 'This is an updated example meeting created via Zoom API.',
}),
}
)
.then((response) => response.json())
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
With these examples, you should be able to get started with the Zoom API using JavaScript. Remember to replace {userId}
, {accessToken}
, and {meetingId}
with the appropriate values for your use case.