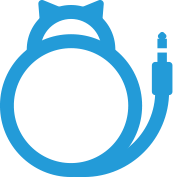
AI Mastering
MusicIntroduction to AIMastering API Docs
If you're looking for a powerful audio mastering tool, AIMastering API is the perfect solution for you. It provides a user-friendly interface and extensive API documentation that can help you build and integrate audio mastering functionality into your applications. In this blog post, we will explore some of the most useful AIMastering API examples and demonstrate how to use them via JavaScript.
API Examples
Authentication
All requests to the API must be authenticated using the Authorization
header and providing a valid API key. Here's an example of how to set the API key in a JavaScript Axios request:
axios.defaults.headers.common['Authorization'] = 'Bearer <YOUR_API_KEY>';
Get Track Analysis
The AIMastering API offers a track analysis feature that enables the user to obtain detailed information about the audio track. This feature can help to inform a mastering engineer of the best approach to take while mastering the track. Here's what the JavaScript code looks like to get track analysis from the AIMastering API:
axios.get('https://api.aimastering.com/v1/analysis/track', {
params: {
fileUrl: '<FILE_URL>',
format: '<FILE_FORMAT>',
privacy: '<PRIVACY>',
sampleRate: '<SAMPLE_RATE>',
bitDepth: '<BIT_DEPTH>',
},
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Get Audio Preview
The AIMastering API can provide a 30-second audio preview of the mastered track. Here's an example of how to use the aupreview
endpoint:
axios.get('https://api.aimastering.com/v1/master/aupreview', {
params: {
style: '<STYLE>',
url: '<FILE_URL>',
format: '<FILE_FORMAT>',
privacy: '<PRIVACY>',
sampleRate: '<SAMPLE_RATE>',
bitDepth: '<BIT_DEPTH>',
},
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Get Mastered Track
Finally, the AIMastering API allows you to download the mastered track directly in your application. Here's how to do it:
axios.get('https://api.aimastering.com/v1/master/file', {
params: {
style: '<STYLE>',
url: '<FILE_URL>',
format: '<FILE_FORMAT>',
privacy: '<PRIVACY>',
sampleRate: '<SAMPLE_RATE>',
bitDepth: '<BIT_DEPTH>',
},
})
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Conclusion
With the AIMastering API, you can integrate powerful audio mastering functionality into your applications in just a few lines of code. In this blog post, we explored some examples of the API in JavaScript. Remember, the key to using the AIMastering API effectively is to read the documentation thoroughly and practice using the API in your applications.