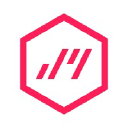
Jamendo
MusicThe Music API by Jamendo is a powerful tool designed for developers who want to integrate a diverse range of music streaming capabilities into their applications. With a comprehensive library of independent music, this API enables users to discover, play, and share tracks from a vast array of genres and artists. By leveraging the extensive database provided, developers can create robust music-related features, enhance user experience, and offer unique services that stand out in the competitive landscape of music applications. The API provides simple endpoints for searching for songs, retrieving album details, and accessing artist information, making it an ideal choice for both novice and experienced developers.
Utilizing the Music API comes with several advantages that enhance music app functionalities. Key benefits include:
- Access to a large collection of independent music tracks.
- Detailed metadata about artists, albums, and tracks for enriched user experiences.
- Flexible search capabilities to discover music based on various filters.
- Integration of streaming features for seamless playback.
- An easy-to-use interface with extensive documentation for rapid development.
Here's a JavaScript code example demonstrating how to call the Music API to search for tracks by a specific artist:
const axios = require('axios');
const searchArtist = async (artistName) => {
const url = `https://api.jamendo.com/v3.0/tracks/?artist=${artistName}&client_id=YOUR_CLIENT_ID`;
try {
const response = await axios.get(url);
const tracks = response.data.results;
tracks.forEach(track => {
console.log(`Title: ${track.name}, Album: ${track.album_title}, Duration: ${track.duration}`);
});
} catch (error) {
console.error('Error fetching data from Jamendo API:', error);
}
};
searchArtist('YourArtistName');
Replace YOUR_CLIENT_ID
with your actual client ID to make API requests. This code snippet highlights how easy it is to fetch and display tracks from the Jamendo music library, offering a solid foundation for building music-oriented applications.