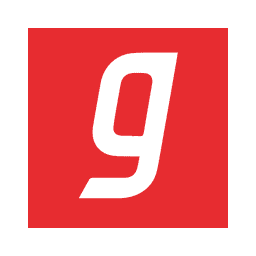
Gaana
MusicExploring GaanaAPI - A Public API for Music Lovers
GaanaAPI is an open-source public API that offers access to a wide range of data related to songs, artists, and albums across various languages. Developers can use these APIs to build apps and services that leverage the Gaana platform and offer an interactive user experience to music lovers.
In this article, we will explore some of the GaanaAPIs and provide examples of how to use them in JavaScript.
Getting Started with GaanaAPI
To get started with GaanaAPI, you first need to create an account on https://gaana.com/developer and obtain an API key. You can view the complete documentation and available endpoints at https://github.com/cyberboysumanjay/GaanaAPI.
Once you have obtained the API key, you can start using the various endpoints available on GaanaAPI.
APIs for Popular Tracks
One of the commonly used APIs is for popular tracks. Let's see an example of how to use this API to fetch popular tracks using JavaScript.
// Importing the required libraries
const axios = require('axios');
// Defining the endpoint URL and API key
const url = `https://cyberboysumanjay.herokuapp.com/gaana/populartracks`;
const apiKey = `your_api_key`;
// Making a GET request with Axios
axios.get(`${url}?key=${apiKey}`)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
This code makes use of the Axios library to make a GET request to the GaanaAPI endpoint for popular tracks. It passes the API key as a query parameter and logs the response data to the console.
APIs for Searching Songs
Another commonly used API is for searching songs. Let's see an example of how to use this API to search for songs using JavaScript.
// Importing the required libraries
const axios = require('axios');
// Defining the endpoint URL and API key
const url = `https://cyberboysumanjay.herokuapp.com/gaana/search`;
const apiKey = `your_api_key`;
// Making a GET request with Axios
axios.get(`${url}?key=${apiKey}&songname=Ishq%20Hai`)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
This code makes use of the Axios library to make a GET request to the GaanaAPI endpoint for searching songs. It passes the API key and the song name as query parameters and logs the response data to the console.
APIs for Album Information
There are various APIs available on GaanaAPI that provide information related to albums and artists. Let's see an example of how to use an endpoint for album information using JavaScript.
// Importing the required libraries
const axios = require('axios');
// Defining the endpoint URL and API key
const url = `https://cyberboysumanjay.herokuapp.com/gaana/albuminfo`;
const apiKey = `your_api_key`;
// Making a GET request with Axios
axios.get(`${url}?key=${apiKey}&albumid=28635`)
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.log(error);
});
This code makes use of the Axios library to make a GET request to the GaanaAPI endpoint for album information. It passes the API key and the album ID as query parameters and logs the response data to the console.
Conclusion
GaanaAPI is a powerful public API that provides access to a wide range of data related to songs, artists, and albums. By leveraging these endpoints, developers can build interactive and engaging music apps and services.
In this article, we explored some of the GaanaAPIs and provided examples of how to use them in JavaScript. We hope this article was helpful in getting you started with GaanaAPI. Happy developing!