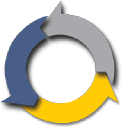
AIS Hub
TransportationExploring the AISHub Public API
AIS (Automatic Identification System) is a widely used technology in the maritime industry that helps in tracking vessels and their movements. AISHub is a global network of AIS receivers that provides live vessel data through their public API.
In this article, we will explore the AISHub Public API and learn how to retrieve live vessel data using JavaScript.
Getting Started
Before we start, we need to obtain an API key from AISHub. The API key can be obtained by registering on their website.
Once we have the API key, we can start making API requests.
Making API Requests
We can use JavaScript's fetch()
method to make API requests. Let's look at an example:
const apiKey = 'YOUR_API_KEY';
const apiUrl = `https://api.aishub.net/ais/v1/vessels/?key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we first define the API key and the API endpoint. We then make a GET request to the endpoint using the fetch()
method. Once we get the response, we convert it to a JSON format using the json()
method. Finally, we log the data to the console.
Parameters
We can use parameters to filter and customize the API response. Let's look at some examples:
Filtering by MMSI
We can filter the response by MMSI (Maritime Mobile Service Identity) number:
const apiKey = 'YOUR_API_KEY';
const mmsi = '123456789';
const apiUrl = `https://api.aishub.net/ais/v1/vessels/?key=${apiKey}&mmsi=${mmsi}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we pass the mmsi
parameter with the desired MMSI number to filter the response.
Limiting Results
We can limit the number of results using the limit
parameter:
const apiKey = 'YOUR_API_KEY';
const limit = 10;
const apiUrl = `https://api.aishub.net/ais/v1/vessels/?key=${apiKey}&limit=${limit}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we pass the limit
parameter with the desired number of results.
Conclusion
In this article, we explored the AISHub Public API and learned how to retrieve live vessel data using JavaScript. We also looked at how to use parameters to filter and customize the API response.
AISHub Public API provides a rich source of vessel data that can be used in various maritime applications.