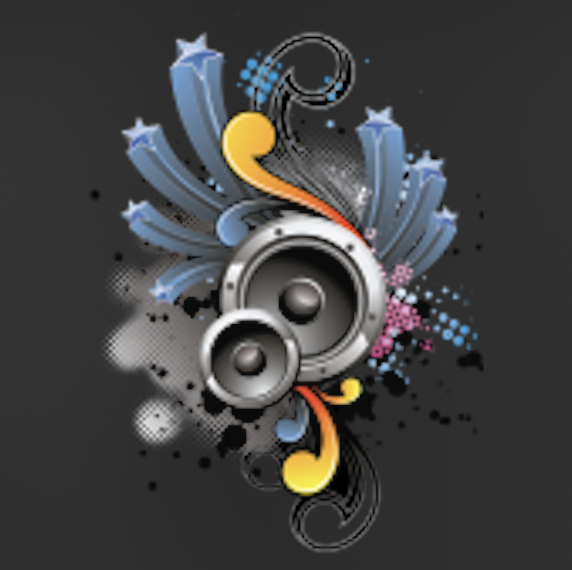
Audio DB API
MusicWith the Audio DB API, you can get albums, artist, specific tracks data, youtube music videos, popularity of an artist and images for 1000s of musicians. For example, on free plan, you can fetch the artist's entire biography. Such as year of birth, style & genre of music, mood of the star, his social media websites, thumbnail, logo, gender, complete description in nearly 6 languages.
📚 Documentation & Examples
Everything you need to integrate with Audio DB API
🚀 Quick Start Examples
// Audio DB API API Example
const response = await fetch('https://www.theaudiodb.com/api_guide.php', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Public API Docs for TheAudioDB
If you are a music lover and want to get access to a lot of information about your favourite artists and their music, then TheAudioDB website is a perfect place to start. TheAudioDB has a massive collection of data related to music, including artist names, album titles, track listings, and more.
The good news is that TheAudioDB also provides a public API that developers can use to get access to this information programmatically. In this article, we will explore the steps required to use TheAudioDB API to retrieve artist data and album information.
Requirements
To get started, you should have a basic understanding of JavaScript and Node.js installed on your machine.
Getting Started
First, you need to sign up for TheAudioDB API by going to https://www.theaudiodb.com/register.php. After completing the registration process, you will receive an API key that you can use to make requests to TheAudioDB API.
To get artist data, use the following code:
const apiKey = 'YOUR_API_KEY';
const artistName = 'Artist Name'; // replace with the name of the artist you want to get data for
const url = `https://www.theaudiodb.com/api/v1/json/${apiKey}/search.php?s=${artistName}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
This code uses the fetch
method to make a GET request to the TheAudioDB API endpoint and passes the artist name and your API key as a parameter. Once you get a response, the data is converted to JSON format, and you can inspect it in the console.
To get album data, use the following code:
const apiKey = 'YOUR_API_KEY';
const albumId = '12345'; // replace with the ID of the album you want to get data for
const url = `https://www.theaudiodb.com/api/v1/json/${apiKey}/album.php?m=${albumId}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
This code is similar to the previous example but uses a different URL and passes the album ID instead of an artist name.
Conclusion
In this article, we have explored TheAudioDB API and looked at examples of how to retrieve artist and album data programmatically using JavaScript. With this knowledge, you can start building your own music applications, leveraging the wealth of data available on the TheAudioDB website.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes