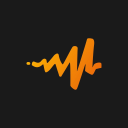
Audiomack
MusicExploring Audiomack's Data API
Audiomack's Data API provides access to their music streaming platform's content and metadata. With this API, you can query for information about artists, songs, albums, playlists, and more. Let's explore some of the APIs by looking at some sample JavaScript code.
Authentication
Before we begin, we need to authenticate ourselves with the API. You can obtain an API key by registering on Audiomack's developer portal. Here's an example of how to authenticate with the API:
const apiKey = 'YOUR_API_KEY';
const authHeader = new Headers({
'Authorization': `Bearer ${apiKey}`
});
Querying for Artists
You can retrieve information about a specific artist by their Audiomack ID. Here's an example of how to do that in JavaScript using fetch:
const artistId = 12345;
const apiUrl = `https://api.audiomack.com/v1/artists/${artistId}`;
fetch(apiUrl, {
headers: authHeader
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Querying for Songs
You can retrieve information about a specific song by its Audiomack ID. Here's an example of how to do that in JavaScript using fetch:
const songId = 67890;
const apiUrl = `https://api.audiomack.com/v1/songs/${songId}`;
fetch(apiUrl, {
headers: authHeader
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Querying for Albums
You can retrieve information about a specific album by its Audiomack ID. Here's an example of how to do that in JavaScript using fetch:
const albumId = 23456;
const apiUrl = `https://api.audiomack.com/v1/albums/${albumId}`;
fetch(apiUrl, {
headers: authHeader
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Querying for Playlists
You can retrieve information about a specific playlist by its Audiomack ID. Here's an example of how to do that in JavaScript using fetch:
const playlistId = 34567;
const apiUrl = `https://api.audiomack.com/v1/playlists/${playlistId}`;
fetch(apiUrl, {
headers: authHeader
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Querying for Trending Content
You can retrieve the top trending content in various categories (songs, albums, playlists, etc.) using the /trending
endpoint. Here's an example of how to query for trending songs in JavaScript using fetch:
const apiUrl = `https://api.audiomack.com/v1/trending/songs`;
fetch(apiUrl, {
headers: authHeader
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
Using Audiomack's Data API, you can query for a wide range of content and metadata related to their music streaming platform. We explored various API calls using JavaScript and I hope this serves as a useful starting point for anyone looking to build on top of Audiomack's platform. Happy coding!