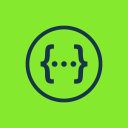
Bandsintown
MusicThe Bandsintown API is designed for artists and enterprises representing artists. It offers read-only access to artist info and artist events. Major end points include artistData, eventData, venueData and offerData. In response you get the name, url, img, facebook page url, tracker counts and upcoming event counts of each artist queried.
📚 Documentation & Examples
Everything you need to integrate with Bandsintown
🚀 Quick Start Examples
// Bandsintown API Example
const response = await fetch('https://app.swaggerhub.com/apis/Bandsintown/PublicAPI/3.0.0', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
A Guide to Using the Bandsintown Public API
Bandsintown is a concert discovery platform that helps music fans track their favorite artists, discover new concerts, and buy tickets. And with the release of the Bandsintown Public API, developers can now integrate concert data into their own applications.
In this guide, we will go over the API basics, including how to authenticate, how to make API requests, and some sample code in JavaScript.
Getting Started
To get started with the Bandsintown Public API, you will need to sign up for an API key by creating a developer account on the SwaggerHub website. Once you have created an account and obtained your API key, you are ready to start making requests.
Authenticating
All requests to the Bandsintown Public API must be made with an API key. To authenticate your requests, you will need to include your API key in the request URL as a query parameter. For example:
https://rest.bandsintown.com/artists/Ed%20Sheeran/events?app_id=YOUR_APP_ID
Replace YOUR_APP_ID
with your actual API key.
Making API Requests
The Bandsintown Public API has endpoints for artists, events, and venues. Here are some examples of API requests you can make in JavaScript:
Get Upcoming Events for an Artist
const artistName = "Ed Sheeran";
const appId = "YOUR_APP_ID";
fetch(`https://rest.bandsintown.com/artists/${encodeURIComponent(artistName)}/events?app_id=${appId}`)
.then(response => response.json())
.then(data => console.log(data));
Replace YOUR_APP_ID
with your actual API key.
Get Events by Date Range
const startDate = "2021-09-01";
const endDate = "2021-09-30";
const appId = "YOUR_APP_ID";
fetch(`https://rest.bandsintown.com/events/search?date=${startDate},${endDate}&app_id=${appId}`)
.then(response => response.json())
.then(data => console.log(data));
Replace YOUR_APP_ID
with your actual API key.
Get Venue Information
const venueId = "1169";
const appId = "YOUR_APP_ID";
fetch(`https://rest.bandsintown.com/venues/${venueId}?app_id=${appId}`)
.then(response => response.json())
.then(data => console.log(data));
Replace YOUR_APP_ID
with your actual API key.
Conclusion
The Bandsintown Public API is a great resource for developers who want to integrate concert data into their applications. We have gone over the basics of the API, including authentication and making API requests, and provided some sample code in JavaScript to help you get started.
If you want to learn more about the Bandsintown Public API, you can check out the official documentation on the SwaggerHub website. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes