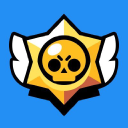
Brawl Stars
Games & ComicsPublic API Documentation for Brawl Stars
Brawl Stars is a popular mobile game with millions of users around the world. If you are looking to develop an application that interacts with Brawl Stars or extract data about the game, you can use the public API offered by the game developers.
This API is available through the Brawl Stars Developer website. It allows you to access various endpoints and retrieve data in JSON format. In this article, we’ll cover the various endpoints available, and some examples of how to use them in JavaScript.
Endpoints
There are several endpoints available through the Brawl Stars API. Here are some of the most commonly used endpoints:
Players
This endpoint allows you to retrieve information about individual Brawl Stars players.
Example endpoint: https://api.brawlstars.com/v1/players/{playerTag}
Brawlers
This endpoint provides information about the different brawlers in the game.
Example endpoint: https://api.brawlstars.com/v1/brawlers
Clubs
This endpoint provides information about clubs (clans) in the game.
Example endpoint: https://api.brawlstars.com/v1/clubs/{clubTag}
Example Code
Now that we know what endpoints are available, let's take a look at some example code in JavaScript.
Get player information
To get information about an individual player, you'll need their player tag. Here's how you can get data about the player with the tag #8CG8LUJ
const fetch = require('node-fetch');
fetch('https://api.brawlstars.com/v1/players/%238CG8LUJ', {
headers: {
'Accept': 'application/json',
'authorization': 'Bearer YOUR_API_TOKEN_HERE'
}
}).then(response => response.json()).then(data => {
console.log(data);
}).catch(err => {
console.error(err);
});
This code uses the node-fetch
package to make a GET request to the players endpoint with the Authorization header set to your API token.
Get club information
To get information about a club, you'll need their club tag. Here's how you can get data about the club with the tag #2RJLGLJ
:
const fetch = require('node-fetch');
fetch('https://api.brawlstars.com/v1/clubs/%232RJLGLJ', {
headers: {
'Accept': 'application/json',
'authorization': 'Bearer YOUR_API_TOKEN_HERE'
}
}).then(response => response.json()).then(data => {
console.log(data);
}).catch(err => {
console.error(err);
});
This code is similar to the player example above, but with the club endpoint instead.
Get brawler information
To get information about all the brawlers, you can make a GET request to the brawlers endpoint:
const fetch = require('node-fetch');
fetch('https://api.brawlstars.com/v1/brawlers', {
headers: {
'Accept': 'application/json',
'authorization': 'Bearer YOUR_API_TOKEN_HERE'
}
}).then(response => response.json()).then(data => {
console.log(data);
}).catch(err => {
console.error(err);
});
This code is similar to the player and club examples above, with the brawlers endpoint used instead.
Conclusion
In this article, we learned about the various endpoints available through the Brawl Stars public API, and saw some examples of how to use them in JavaScript. Now that you know how to use these endpoints, you can start building your own application or extracting data about the game. Happy coding!