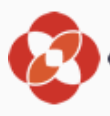
Connexun
NewsConnexun News API - A Comprehensive Guide with JavaScript Examples
Connexun News API is an excellent web service that provides the latest news, articles, and blog posts from various news sources and blogs. This powerful API is perfect for developers who want to create apps that rely on real-time news data.
In this blog post, we're going to take a detailed look at how the Connexun News API works and how you can use it in your JavaScript applications. We'll cover everything from setting up the API to retrieving data and parsing it.
Getting Started
To use the Connexun News API, you'll need to sign up for an account and obtain an API key. Once you have your key, you can access the API endpoints using HTTP requests.
Here's a basic example of how to retrieve the latest news using the Connexun News API with JavaScript.
const endpoint = 'https://api.connexun.com/news-api/v2';
const key = 'your-api-key';
fetch(`${endpoint}/articles?country=US&apiKey=${key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're using the fetch
function to send an HTTP GET request to the Connexun News API. We're retrieving the latest news articles from the United States and passing in our API key as a parameter.
Once we receive a response, we're parsing the JSON data and logging it to the console. If there's an error, we're logging it to the console as well.
API Endpoints
The Connexun News API provides several endpoints to retrieve news data. Here are some examples of how to use each endpoint with JavaScript.
Top Headlines
The top headlines endpoint returns the top headlines from various news sources.
fetch(`${endpoint}/top-headlines?country=US&category=health&apiKey=${key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're retrieving the top health headlines from the United States.
Everything
The everything endpoint returns all news articles that match the search query.
fetch(`${endpoint}/everything?q=bitcoin&apiKey=${key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're retrieving all news articles related to bitcoin.
Sources
The sources endpoint returns all news sources.
fetch(`${endpoint}/sources?apiKey=${key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're retrieving all news sources.
Parsing API Data
The data retrieved from the Connexun News API is in JSON format. To work with this data, we'll need to parse it into an object using JavaScript.
fetch(`${endpoint}/articles?country=US&apiKey=${key}`)
.then(response => response.json())
.then(data => {
data.articles.forEach(article => {
console.log(article.title);
console.log(article.description);
console.log(article.url);
console.log(article.urlToImage);
console.log(article.publishedAt);
})
})
.catch(error => console.error(error));
In this example, we're retrieving the latest news articles from the United States and parsing the JSON data. We're then using a forEach
loop to iterate through each article object and logging specific properties such as the title, description, URL, image, and publish date to the console.
Conclusion
The Connexun News API is a powerful tool for developers who want to create real-time news applications. With endpoints for top headlines, everything, and sources, retrieving and parsing news data is now easier and more efficient than ever.
Using JavaScript, you can quickly integrate the Connexun News API into your applications and create engaging news experiences for your users.