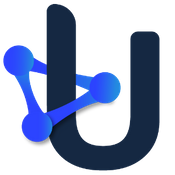
Contextual Web
NewsSearch from 100k news sources to find news feeds, posts, articles, and blogs on the topic of your choice using ContextualWeb. By integrating with RapidAPI, you can obtain your API key and make a GET request within seconds. ContexualWeb has one of the most advanced search algorithms on the internet, which helps you find what you are looking for precisely and fast. They respect your privacy by employing the highest privacy protection; hence, your search history or data is never available to someone else.
📚 Documentation & Examples
Everything you need to integrate with Contextual Web
🚀 Quick Start Examples
// Contextual Web API Example
const response = await fetch('https://usearch.com/news-api/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the Usearch News API in JavaScript
Usearch offers a powerful News API that allows developers to retrieve news articles from multiple sources and languages. In this tutorial, we will explore how to use the Usearch News API in JavaScript and provide some example code to help you get started.
Getting started
To use the Usearch News API, you will need an API key. If you don't have one already, sign up for a free account on the Usearch website and generate an API key from the dashboard.
Example code
Fetching headlines
const API_KEY = 'YOUR_API_KEY';
const url = `https://api.usearch.com/v2/top-headlines?country=us&pageSize=10&apiKey=${API_KEY}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code fetches the top headlines from the US, limited to 10 articles per request. To use this code, replace YOUR_API_KEY
with your actual API key.
Searching for articles
const API_KEY = 'YOUR_API_KEY';
const searchQuery = 'bitcoin';
const url = `https://api.usearch.com/v2/everything?q=${searchQuery}&apiKey=${API_KEY}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code fetches all articles that match the search query bitcoin
. To use this code, replace YOUR_API_KEY
with your actual API key and bitcoin
with your desired search term.
Filtering articles by language
const API_KEY = 'YOUR_API_KEY';
const searchQuery = 'apple';
const language = 'en';
const url = `https://api.usearch.com/v2/everything?q=${searchQuery}&language=${language}&apiKey=${API_KEY}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code fetches all English-language articles that match the search query apple
. To use this code, replace YOUR_API_KEY
with your actual API key, apple
with your desired search term, and en
with your desired language code.
Conclusion
In this post, we covered how to use the Usearch News API in JavaScript with some example code snippets. The Usearch News API is a great tool for developers who want to integrate the latest news into their applications. We hope this tutorial has provided you with a solid foundation to start exploring the Usearch News API on your own.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes