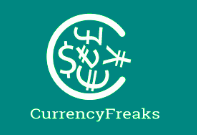
CurrencyFreaks API
Currency ExchangeUsing the CurrencyFreaks API to fetch currency exchange rates
Are you looking to build an app that requires up-to-date currency exchange rates? Look no further than the CurrencyFreaks API. In this blog post, we'll walk through how to use the CurrencyFreaks API to get the latest currency exchange rates.
API Authorization
To use the CurrencyFreaks API, you'll need to sign up for an API key. You can sign up for a free account to get your API key.
Once you have your API key, you'll need to include it in the headers of your API requests. Here's an example of how to do that:
curl -H "apikey: YOUR_API_KEY" https://api.currencyfreaks.com/latest
Fetching Currency Exchange Rates
To fetch the latest currency exchange rates, you can make a request to the latest
endpoint of the CurrencyFreaks API. Here's an example of how to do that in JavaScript:
fetch('https://api.currencyfreaks.com/latest?apikey=YOUR_API_KEY')
.then(response => response.json())
.then(data => console.log(data));
This will fetch the latest currency exchange rates as a JSON object. You can then use this data in your app as needed.
Specifying Base Currency
By default, the CurrencyFreaks API returns exchange rates based on the USD. If you'd like to specify a different base currency, you can do so by adding a base
parameter to your request. Here's an example:
fetch('https://api.currencyfreaks.com/latest?apikey=YOUR_API_KEY&base=EUR')
.then(response => response.json())
.then(data => console.log(data));
This will fetch the latest currency exchange rates based on the EUR.
Specifying Quote Currencies
By default, the CurrencyFreaks API returns exchange rates for all available currencies. If you'd like to specify a subset of quote currencies, you can do so by adding a symbols
parameter to your request. Here's an example:
fetch('https://api.currencyfreaks.com/latest?apikey=YOUR_API_KEY&symbols=EUR,JPY')
.then(response => response.json())
.then(data => console.log(data));
This will fetch the latest currency exchange rates for the EUR and JPY.
Conclusion
The CurrencyFreaks API is a great option for fetching up-to-date currency exchange rates. With just a few lines of code in JavaScript, you can access the latest exchange rates and use them in your app. Happy coding!