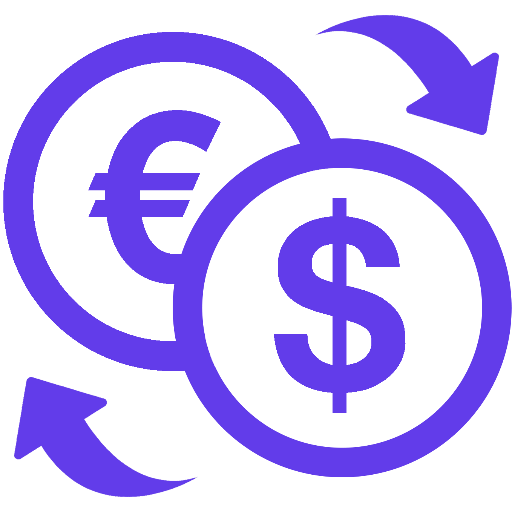
CurrenyAPI
Currency ExchangeIntroduction to Currency API
Currency API is a free, simple, and lightweight API that provides real-time exchange rates for various currencies around the world. It supports over 160 currencies and is constantly updated to reflect the latest market data.
In this article, we'll walk you through the various API endpoints offered by Currency API and provide sample code in JavaScript to help you get started.
Getting Started
Before you can use the Currency API, you'll need to sign up for a free API key. You can do this by visiting the Currency API website and clicking on the "Get API Key" button.
Once you've obtained your API key, you can use it to make requests to the various endpoints offered by the Currency API.
Retrieving Exchange Rates
The Currency API provides several endpoints for retrieving exchange rates that can be used to calculate the conversion rate between two currencies. Here's an example of how to retrieve the exchange rate between the USD and EUR using JavaScript:
fetch('https://api.currencyapi.net/v1/rates?key=YOUR_API_KEY&base=USD')
.then(response => response.json())
.then(data => console.log(data.rates.EUR));
In this example, we're using the fetch
function to make a GET request to the Currency API's /v1/rates
endpoint. We're passing our API key as a URL parameter and specifying that we want the exchange rate for USD as the base currency.
The response from the API is returned as a JSON object, which we can access using the response.json()
method. In the final step, we're logging the exchange rate for EUR to the console.
Converting Currency
In addition to retrieving exchange rates, the Currency API also provides an endpoint for converting currency. Here's an example of how to convert 100 USD to EUR using JavaScript:
fetch('https://api.currencyapi.net/v1/convert?key=YOUR_API_KEY&q=100-USD-to-EUR')
.then(response => response.json())
.then(data => console.log(data.result));
In this example, we're using the /v1/convert
endpoint to convert 100 USD to EUR. We're passing our API key as a URL parameter and specifying the conversion query as 100-USD-to-EUR
.
The response from the API is returned as a JSON object, which we can access using the response.json()
method. In the final step, we're logging the converted value to the console.
Conclusion
The Currency API is a powerful tool for retrieving and converting real-time exchange rates. In this article, we've shown you how to use the API with JavaScript to retrieve exchange rates and perform currency conversions.
If you're interested in learning more about the Currency API, be sure to check out the official documentation for a complete list of endpoints and examples in various programming languages.