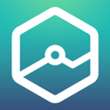
Diabetes
HealthIntroduction
The http://predictbgl.com/api/ API provides a platform to predict blood glucose levels. In this blog, we will explore some of the possible API examples in JavaScript.
Getting Started
Before we dive into the examples, we need to obtain an API key from the http://predictbgl.com/api/ website. Once we have the API key, we can use it to authenticate and access the available API endpoints.
Authentication
To authenticate ourselves, we need to pass our API key in the header of the request. Below is the example code snippet to authenticate using JavaScript.
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer <API-KEY>'
};
Here, we have added the API key to the Authorization
header, which is prefixed by 'Bearer '
.
API Endpoints
The http://predictbgl.com/api/ website provides different endpoints to make predictions, update, delete, and retrieve a user's data.
Predictions
We can predict blood glucose levels for a user by calling the /predict
endpoint. To make a prediction, we need to pass the user's blood glucose readings and carb intake data in the request body.
Below is the example code snippet to make a prediction using JavaScript.
const data = {
"in": [],
"bg": [],
"carb": []
};
const requestOptions = {
method: 'POST',
headers: headers,
body: JSON.stringify(data),
redirect: 'follow'
};
fetch("http://predictbgl.com/api/v1/predict", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Here, we have created a data
object and passed the user's blood glucose readings, carb intake data and insulin dosage (optional). In the requestOptions
, we have added the method
, headers
, body
, and redirect
preferences for our POST request. We will receive a prediction in the response.
Update User Data
We can update a user's data by calling the /update
endpoint. To update the user's data, we need to pass the user's id and desired changes (which can be insulin sensitivity, target range, and profile name) in the request body.
Below is the example code snippet to update a user's data using JavaScript.
const data = {
"id": "<USER-ID>",
"insulinSensitivity": 70,
"targetHigh": 180,
"targetLow": 80,
"profileName": "my new profile"
};
const requestOptions = {
method: 'PUT',
headers: headers,
body: JSON.stringify(data),
redirect: 'follow'
};
fetch("http://predictbgl.com/api/v1/update", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Here, we have created a data
object and passed the user's id and desired changes (which are insulin sensitivity, target range, and profile name) in the requestOptions
. We will receive the updated user's data in the response.
Delete User Data
We can delete a user's data by calling the /delete
endpoint. To delete the user's data, we need to pass the user's id in the request body.
Below is the example code snippet to delete a user's data using JavaScript.
const data = {
"id": "<USER-ID>"
};
const requestOptions = {
method: 'DELETE',
headers: headers,
body: JSON.stringify(data),
redirect: 'follow'
};
fetch("http://predictbgl.com/api/v1/delete", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Here, we have created a data
object and passed the user's id to the requestOptions
. We will receive a success message in the response.
Retrieve User Data
We can retrieve a user's data by calling the /get
endpoint. To retrieve the user's data, we need to pass the user's id in the request body.
Below is the example code snippet to retrieve a user's data using JavaScript.
const data = {
"id": "<USER-ID>"
};
const requestOptions = {
method: 'POST',
headers: headers,
body: JSON.stringify(data),
redirect: 'follow'
};
fetch("http://predictbgl.com/api/v1/get", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
Here, we have created a data
object and passed the user's id to the requestOptions
. We will receive the user's data in the response.
Conclusion
In this blog, we have covered some of the possible API examples in JavaScript to use the http://predictbgl.com/api/ API. These examples will help you to implement the API for your projects. However, it is advisable to refer to the API documentation for detailed information.