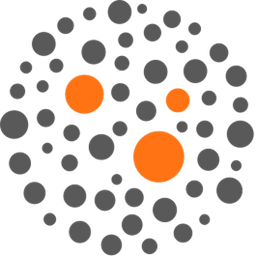
Nutritionix
HealthIntroduction to Using the Public API Docs from Nutritionix
If you are interested in developing an application that uses nutrition information, the Nutritionix public API is a great resource. The API provides access to a large database of nutritional information for foods, brands, and restaurants.
In this tutorial, we will show you how to use the Nutritionix public API docs and provide example code in JavaScript to help you get started.
Step 1: Create an Account
To use the Nutritionix API, you need to create an account on their website. Once you have created an account, you will need to create an API Application. This will provide you with an API Key and Secret Key that you will use to authenticate your requests.
Step 2: Authentication
Before we can make any requests to the Nutritionix API, we need to authenticate ourselves using our API Key and Secret Key. In our example code, we will be using the axios library to make our requests.
const axios = require('axios');
const APP_ID = 'YOUR_APP_ID';
const APP_KEY = 'YOUR_APP_KEY';
axios.defaults.headers.common['x-app-id'] = APP_ID;
axios.defaults.headers.common['x-app-key'] = APP_KEY;
Step 3: Search for a Food Item
Now that we have authenticated ourselves, we can start making requests to the Nutritionix API to search for food items. In this example, we will search for a food item called "apple".
axios.get(`https://api.nutritionix.com/v1_1/search/apple?results=0%3A20&fields=item_name%2Citem_id%2Cbrand_name%2Cnf_calories%2Cnf_total_fat`, {
headers: {
'x-app-id': APP_ID,
'x-app-key': APP_KEY,
}
})
.then(response => {
console.log(response.data.hits);
})
.catch(error => {
console.log(error);
});
Step 4: Retrieve Nutrition Information for a Food Item
Now that we have found a food item, we can retrieve the nutrition information for that item. In this example, we will retrieve the nutrition information for a food item with an item id of "513fc9cb673c4fbc26004461".
axios.get(`https://api.nutritionix.com/v1_1/item?id=513fc9cb673c4fbc26004461`, {
headers: {
'x-app-id': APP_ID,
'x-app-key': APP_KEY,
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Conclusion
Using the Nutritionix public API is a great way to access nutrition information for a wide range of foods, brands, and restaurants. With the example code we have provided, you can get started with using the API in your own application. Remember to always use your own API Key and Secret Key, and to be mindful of any rate limits or usage restrictions that may apply.