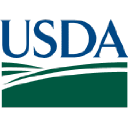
USDA Nutrients
HealthUsing the USDA Food Data Central API with JavaScript
The USDA Food Data Central API provides access to a variety of food and nutrient data, including information on over 300,000 foods. In this post, we'll explore how to use this API with JavaScript.
Getting an API Key
Before we can start using the API, we'll need to get an API key. To do this, we'll need to create an account on the USDA Food Data Central website and request an API key. Once we have our API key, we can start using the API.
Making Requests
The API documentation provides information on the available endpoints and parameters. To make a request, we'll need to include our API key in the request URL, along with any other parameters we want to use.
const apiKey = 'YOUR_API_KEY';
const query = 'chicken';
fetch(`https://api.nal.usda.gov/fdc/v1/foods/search?api_key=${apiKey}&query=${query}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the fetch
function to make a request to the /foods/search
endpoint. We're passing our API key and a query string parameter for the search term 'chicken'. Once we receive the response, we're parsing the JSON data and logging it to the console.
Filtering Results
The API also allows us to filter the results using various parameters, such as food category or nutrient information. Here's an example of how we can filter by food category:
const apiKey = 'YOUR_API_KEY';
const categoryId = 4; // Category ID for 'Dairy and Egg Products'
fetch(`https://api.nal.usda.gov/fdc/v1/foods/search?api_key=${apiKey}&category=${categoryId}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the category
parameter to filter the search results to only include foods from the 'Dairy and Egg Products' category.
Retrieving Nutrient Information
One of the main benefits of the USDA Food Data Central API is the ability to retrieve nutrient information for individual foods. We can do this by using the fdcId
parameter to specify the ID of the food we're interested in, and including the nutrients
parameter to specify which nutrient information we want to retrieve.
const apiKey = 'YOUR_API_KEY';
const fdcId = 167778; // ID for 'Chicken Breast'
fetch(`https://api.nal.usda.gov/fdc/v1/foods/${fdcId}/nutrients?api_key=${apiKey}&nutrients=203`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we're using the fdcId
parameter to retrieve nutrient information for chicken breast. We've also included the nutrients
parameter to specify that we only want to retrieve information about protein (nutrient ID 203).
Conclusion
The USDA Food Data Central API provides a wealth of food and nutrient information that can be incredibly useful for a variety of applications. By using JavaScript, we can interact with the API and filter, search, and retrieve the information we need. Get creative and see what kind of food-related applications you can build!