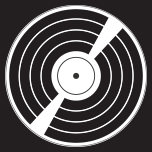
Discogs
MusicExploring the Discogs Public API with JavaScript
Discogs is a popular music marketplace where users can buy and sell vinyl, CDs, and other types of music media. Discogs also offers a public API that allows developers to access and interact with the marketplace's data. In this blog post, we will explore the Discogs Public API and provide some examples of how to use it in JavaScript.
Getting Started
Before we can start using the Discogs Public API, we need to obtain an API key. To do this, we need to register an account on the Discogs website and create an application to get a key. Once we have our key, we can start making requests to the API.
Making API Requests in JavaScript
To make API requests in JavaScript, we can use the built-in fetch function. The fetch function returns a Promise that resolves to the response from the API server. We can then use methods like .json() to extract the data from the response.
Here is an example of making a request to get information about a specific release:
fetch('https://api.discogs.com/releases/1', {
headers: { 'Authorization': 'Discogs key=[YOUR_KEY],secret=[YOUR_SECRET]' }
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are using the fetch function to get information about the release with ID 1. We are also including our API key in the headers of the request. The response is then converted to a JSON object and logged to the console.
Searching for Releases
One of the most common use cases for the Discogs API is to search for releases based on certain criteria. Here is an example of using the search API to find all releases by a specific artist:
fetch('https://api.discogs.com/database/search?q=artist:"Radiohead"&type=release&per_page=10', {
headers: { 'Authorization': 'Discogs key=[YOUR_KEY],secret=[YOUR_SECRET]' }
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are searching for releases where the artist name contains the string "Radiohead". We are also specifying that we only want to search for release types, and that we want a maximum of 10 results per page.
Conclusion
The Discogs Public API provides a wealth of information about music releases. With JavaScript, we can easily make requests to the API and process the data that is returned. Hopefully, these examples have given you a good starting point for using the Discogs API in your own projects.