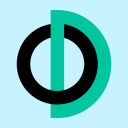
Dota 2
Games & ComicsThe OpenDota API provides Dota 2 related data including advanced match data extracted from match replays.
📚 Documentation & Examples
Everything you need to integrate with Dota 2
🚀 Quick Start Examples
// Dota 2 API Example
const response = await fetch('https://docs.opendota.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring OpenDota Public API
OpenDota Public API provides free access to the extensive dataset of Dota 2 matches, players, heroes, items, and more. In this tutorial, we will explore the various endpoints of the OpenDota API and see how to use them with JavaScript.
Getting started
Before we start making API calls, we need to get an API key from the OpenDota website. Go to https://www.opendota.com/ and sign up for a free account. Once you have an account, navigate to the API documentation page to see the full list of API endpoints.
Retrieving hero data
Let's start by retrieving data for a specific hero. In this example, we will use the hero ID for Earthshaker.
const request = require('request');
const options = {
url: 'https://api.opendota.com/api/heroes/earthshaker',
method: 'GET',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
};
request(options, (error, response, body) => {
if (!error && response.statusCode == 200) {
const data = JSON.parse(body);
console.log(data);
} else {
console.error(error);
}
});
This will make a GET request to the OpenDota API with the hero ID as a parameter. The API will return a JSON response with information about the hero, including their name, abilities, and stats.
Retrieving match data
Next, let's retrieve data for a specific match. In this example, we will use the match ID for a recent professional match.
const request = require('request');
const options = {
url: 'https://api.opendota.com/api/matches/5905070268',
method: 'GET',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
};
request(options, (error, response, body) => {
if (!error && response.statusCode == 200) {
const data = JSON.parse(body);
console.log(data);
} else {
console.error(error);
}
});
This will make a GET request to the OpenDota API with the match ID as a parameter. The API will return a JSON response with information about the match, including the players, heroes, and events.
Retrieving player data
Finally, let's retrieve data for a specific player. In this example, we will use the player ID for a top-ranked player.
const request = require('request');
const options = {
url: 'https://api.opendota.com/api/players/86745912',
method: 'GET',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
};
request(options, (error, response, body) => {
if (!error && response.statusCode == 200) {
const data = JSON.parse(body);
console.log(data);
} else {
console.error(error);
}
});
This will make a GET request to the OpenDota API with the player ID as a parameter. The API will return a JSON response with information about the player, including their name, rank, and match history.
Conclusion
OpenDota Public API provides a comprehensive dataset for Dota 2 enthusiasts and developers. By using JavaScript to make API requests, you can easily retrieve and analyze data for heroes, matches, and players. With this code as a starting point, you can create your own tools and visualizations to explore the vast world of Dota 2. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes