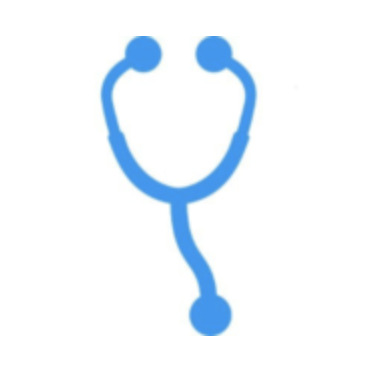
EndlessMedical API
HealthExploring the Endless Medical API with JavaScript
Endless Medical offers an API that allows developers to access a vast database of medical information. This API is great for creating healthcare apps, machine learning models, and more. In this post, we'll explore the Endless Medical API and provide examples of how to use it with JavaScript.
Getting Started with the Endless Medical API
Before we can start using the Endless Medical API, we need to register for an account and obtain an API key. To register for an Endless Medical account, visit the Endless Medical Sandbox API website and click the "Get API Key" button. Fill in your details and submit the form. You'll get an API key which should be kept safe.
Making API Requests with JavaScript
We can make API requests to the Endless Medical API with the Fetch API in JavaScript. The Fetch API provides a modern and easy to use interface for making network requests, and it's supported in all modern browsers.
For our first example, we'll search the Endless Medical database for all doctors with the name "Smith". We can do this using the search/doctors
endpoint.
const apiKey = 'YOUR_API_KEY';
fetch(`https://api.endlessmedical.com/v1/search/doctors?q=smith&api_key=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above code, we're making a GET
request to the search/doctors
endpoint with a search term of "smith" and our API key. We're also using the .json()
method to parse the response data.
The response data will be an array of doctor objects that look like this:
[
{
"id": "65749dd6b8ca57e5fe9de02c5a92a42c",
"first_name": "John",
"last_name": "Smith",
"gender": "Male",
"address": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "12345",
"phone": "(555) 555-5555",
"fax": "(555) 555-5555",
"npi": "1234567890",
"specialty": "Family Medicine",
"degree": "MD",
"board_certified": false,
"languages": [
"English",
"Spanish"
]
}
]
We can then use this data to display a list of search results, or use it in any other way we see fit.
Conclusion
In this post, we've explored the Endless Medical API and demonstrated how to use it with JavaScript. We've used the Fetch API to make network requests and handled the response data with .json()
. With this knowledge, you can start building your own healthcare apps, machine learning models, and more.