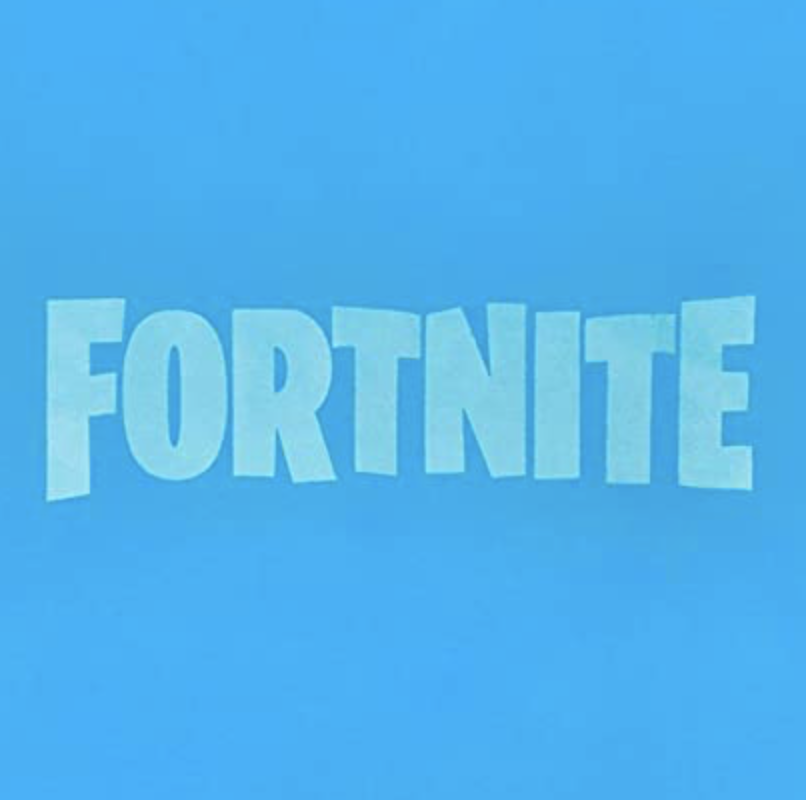
Fortnite Tracker API
Games & ComicsWe provide this API as an easy way for developers to get information from Fortnite. This is a great API for bots (twitch, discord, etc), tools and research projects. 1 request per 2 seconds. Contact us for an increased limit. We do not offer any support or programming support. Do not contact us for help. This API is provided as-is and we reserve the right to ban you, reduce/increase the rate limits, or disable it completely. Requests will return remaining throttle left in the headers. This is a free API, we do not charge for using it, nor do we charge for increasing your limit, but we do need to verify you are not using it for nefarious reasons. We respect Epic's servers and Game.
📚 Documentation & Examples
Everything you need to integrate with Fortnite Tracker API
🚀 Quick Start Examples
// Fortnite Tracker API API Example
const response = await fetch('https://tracker.gg/developers', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the Fortnite Tracker API with JavaScript
If you're a developer looking to tap into Fortnite's data, the Fortnite Tracker API is an excellent resource. With the release of Season 7, the API now includes information about Creative mode in addition to Battle Royale and Save the World.
This blog post will cover how to use the Fortnite Tracker API with JavaScript, with example code snippets.
Getting Started
Before we can start making requests to the API, we need to create an account and get an API key. Head to https://fortnitetracker.com/site-api and sign up for an account. Once you've done that, navigate to the "API Key" tab and generate a new API key.
Now that we have an API key, let's start making requests!
Examples
Finding a player's stats
// Include the axios library
const axios = require('axios');
// Set the API endpoint and parameters
const API_ENDPOINT = 'https://api.fortnitetracker.com/v1/profile';
const headers = {
'TRN-Api-Key': 'YOUR_API_KEY_HERE',
};
const username = 'Ninja';
const platform = 'pc';
// Make the request
axios.get(`${API_ENDPOINT}/${platform}/${username}`, { headers })
.then((response) => {
const stats = response.data.lifeTimeStats;
console.log(`${username} has ${stats[7].value} total kills`);
})
.catch((error) => {
console.error(error);
});
This code uses Axios to make a GET request to the /profile
endpoint, passing in the player's platform and username as parameters. The response data includes a lifeTimeStats
array, which contains various stats about the player's lifetime performance. In this example, we're logging the player's total kills.
Finding a player's match history
// Include the axios library
const axios = require('axios');
// Set the API endpoint and parameters
const API_ENDPOINT = 'https://api.fortnitetracker.com/v1/powerrankings';
const headers = {
'TRN-Api-Key': 'YOUR_API_KEY_HERE',
};
const username = 'Ninja';
const platform = 'pc';
// Make the request
axios.get(`${API_ENDPOINT}/${platform}/${username}`, { headers })
.then((response) => {
const matches = response.data.lifeTimeStats;
console.log(`${username} has played ${matches.length} matches`);
})
.catch((error) => {
console.error(error);
});
This code is similar to the previous example, but instead of querying the player's lifetime stats, we're querying their recent match history. The response data includes an array of matches with information about each one. In this example, we're logging the number of matches the player has played.
Finding a player's leaderboard rank
// Include the axios library
const axios = require('axios');
// Set the API endpoint and parameters
const API_ENDPOINT = 'https://api.fortnitetracker.com/v1/leaderboards';
const headers = {
'TRN-Api-Key': 'YOUR_API_KEY_HERE',
};
const platform = 'pc';
const region = 'global';
// Make the request
axios.get(`${API_ENDPOINT}/${platform}/${region}`, { headers })
.then((response) => {
const leaderboard = response.data.entries;
const ninja = leaderboard.find((entry) => entry.epicUserHandle === 'Ninja');
console.log(`${ninja.epicUserHandle} is ranked ${ninja.rank} globally`);
})
.catch((error) => {
console.error(error);
});
This code queries the leaderboard for the global platform and logs Ninja's rank. The response data includes an array of leaderboard entries, with information about each player's stats and rank. In this example, we're finding the entry for Ninja and logging his rank.
Conclusion
The Fortnite Tracker API provides a wealth of data for developers to tap into. With the examples provided in this post, you should be able to start making requests to the API and get a feel for the data available. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes