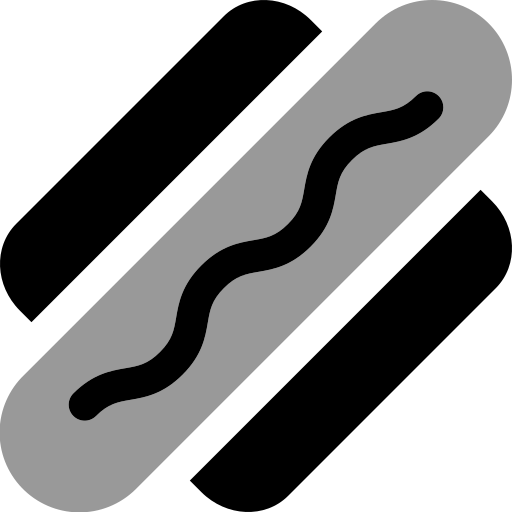
Frankfurter
Currency ExchangeHow to Use Frankfurter API
Frankfurter API is a public API that provides currency exchange rate data for free. You can easily use this API in your JavaScript project to get real-time exchange rates.
How to Access Frankfurter API
To access Frankfurter API, you need to make an HTTP request to the API endpoint using fetch()
. Here is the API endpoint:
https://api.frankfurter.app/latest
You can add query parameters to this endpoint to get exchange rates for a specific date or for specific currencies. For example, to get the exchange rates for USD and EUR for today, you can use this endpoint:
https://api.frankfurter.app/latest?from=USD&to=EUR
How to Get Exchange Rates
To get exchange rates from Frankfurter API, you can use the following code:
const url = 'https://api.frankfurter.app/latest?from=USD&to=EUR';
fetch(url)
.then(response => response.json())
.then(data => {
const rate = data.rates.EUR;
console.log(rate);
});
In this code, we are making a fetch()
request to the Frankfurter API using the URL that we constructed above. We are then using .json()
to parse the response and get the data as a JavaScript object. Finally, we are accessing the EUR
rate by using data.rates.EUR
.
How to Get Historical Exchange Rates
To get historical exchange rates from Frankfurter API, you can use the following code:
const date = '2022-01-01';
const url = `https://api.frankfurter.app/${date}?from=USD&to=EUR`;
fetch(url)
.then(response => response.json())
.then(data => {
const rate = data.rates.EUR;
console.log(rate);
});
In this code, we are specifying a date in ISO format (YYYY-MM-DD
) and constructing the API endpoint URL using that date. We are then fetching the data and accessing the EUR
rate as before.
Conclusion
Frankfurter API is a powerful tool that provides real-time and historical exchange rates. With just a few lines of JavaScript code, you can easily incorporate this API into your project and get the latest exchange rates.