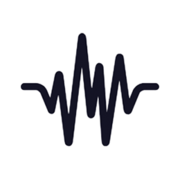
FreeSound
MusicAn Introduction to the FreeSound API
If you're interested in accessing a massive database of sounds and audio clips, the FreeSound API is worth checking out. This public API provides a wealth of data and information about a variety of different sounds, ranging from natural noises to musical recordings and more.
Getting Started with the FreeSound API
Before we dive into some example code, here's a quick overview of how you can get started with the FreeSound API:
- First, you need to register for a FreeSound account. Once you've got your account set up, you can get an API key by going to the "Developers" section of the FreeSound website.
- Once you have your API key, you can start making requests to the FreeSound API from your own code.
Making API Requests in JavaScript
To help you get started with the FreeSound API, here are some example code snippets in JavaScript that show you how to make various types of requests:
Searching for Sounds
To search for sounds in the FreeSound API, you can use the following code:
const apiKey = 'YOUR_API_KEY_GOES_HERE';
const query = 'piano';
const url = `https://freesound.org/apiv2/search/text/?query=${query}&token=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code uses the fetch
function to make a request to the API with a search query for "piano". The response data is returned as a JSON object that can be accessed in the then
callback.
Accessing Sound Details
To access details about a specific sound in the FreeSound API, you can use the following code:
const apiKey = 'YOUR_API_KEY_GOES_HERE';
const soundId = 1234;
const url = `https://freesound.org/apiv2/sounds/${soundId}/?token=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code makes a request to the API for the sound with the ID of 1234. Again, the response data is returned as a JSON object that can be accessed in the then
callback.
Accessing Sound Analyses
Finally, you can also access detailed analyses of sounds in the FreeSound API using the following code:
const apiKey = 'YOUR_API_KEY_GOES_HERE';
const soundId = 1234;
const url = `https://freesound.org/apiv2/sounds/${soundId}/analysis/?token=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This code requests the detailed analysis of the sound with the ID of 1234. Again, the response data is returned as a JSON object that can be accessed in the then
callback.
Conclusion
The FreeSound API is a powerful and versatile tool for accessing a wide range of sounds and audio data. With these code examples in JavaScript, you should be able to get started with making your own requests and exploring the many features of this impressive API.