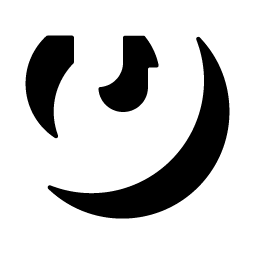
Genius
MusicAccessing the Genius API with JavaScript
If you're looking to integrate the Genius API into your JavaScript project, then you're in luck. The Genius API is a public API that allows developers to access detailed information on songs, musical artists, and lyrics.
To get started, you'll need an API key. You can request a Genius API key by signing up for a free developer account on the Genius Developer Platform. Once you have your key, you're ready to start making API requests.
Before we dive into the code examples, it's important to know that the Genius API uses OAuth 2.0 for authentication. This means that you'll need to provide a valid access token with each API request. There are a few different ways to obtain an access token, but for simplicity's sake, we'll use the client ID and client secret approach.
Here's an example of how to obtain an access token:
const clientId = '<your client ID>';
const clientSecret = '<your client secret>';
const authUrl = 'https://api.genius.com/oauth/token';
const authHeaders = {
'Content-Type': 'application/x-www-form-urlencoded',
Authorization: `Basic ${btoa(`${clientId}:${clientSecret}`)}`,
};
const authData = {
grant_type: 'client_credentials',
};
const authToken = await fetch(authUrl, {
method: 'POST',
headers: authHeaders,
body: new URLSearchParams(authData),
}).then((response) => response.json()).then((data) => data.access_token);
Once you have an access token, you can start making API requests. Here are some examples of how to use the Genius API with JavaScript:
Searching for Songs
const searchTerm = 'Kendrick Lamar';
const searchUrl = `https://api.genius.com/search?q=${encodeURIComponent(searchTerm)}`;
const searchHeaders = {
Authorization: `Bearer ${authToken}`,
};
const searchResults = await fetch(searchUrl, {
headers: searchHeaders,
}).then((response) => response.json()).then((data) => data.response.hits);
console.log(searchResults);
This code will search the Genius API for songs that match the search term "Kendrick Lamar". The results will be logged to the console.
Getting Song Information
const songId = 378195;
const songUrl = `https://api.genius.com/songs/${songId}`;
const songHeaders = {
Authorization: `Bearer ${authToken}`,
};
const songData = await fetch(songUrl, {
headers: songHeaders,
}).then((response) => response.json()).then((data) => data.response.song);
console.log(songData);
This code will retrieve detailed information on the song with ID 378195. The results will be logged to the console.
Getting Artist Information
const artistId = 1421;
const artistUrl = `https://api.genius.com/artists/${artistId}`;
const artistHeaders = {
Authorization: `Bearer ${authToken}`,
};
const artistData = await fetch(artistUrl, {
headers: artistHeaders,
}).then((response) => response.json()).then((data) => data.response.artist);
console.log(artistData);
This code will retrieve detailed information on the artist with ID 1421. The results will be logged to the console.
Getting Lyrics
const songId = 378195;
const songUrl = `https://api.genius.com/songs/${songId}`;
const songHeaders = {
Authorization: `Bearer ${authToken}`,
};
const songData = await fetch(songUrl, {
headers: songHeaders,
}).then((response) => response.json()).then((data) => data.response.song);
const lyricsUrl = songData.url + '/lyrics';
const lyricsData = await fetch(lyricsUrl).then((response) => response.text());
console.log(lyricsData);
This code will retrieve the lyrics for the song with ID 378195. The lyrics will be logged to the console.
Conclusion
In this blog post, we learned how to use the Genius API with JavaScript. We covered how to obtain an access token, search for songs, retrieve song and artist information, and retrieve lyrics. With this knowledge, you can start integrating the Genius API into your JavaScript projects.