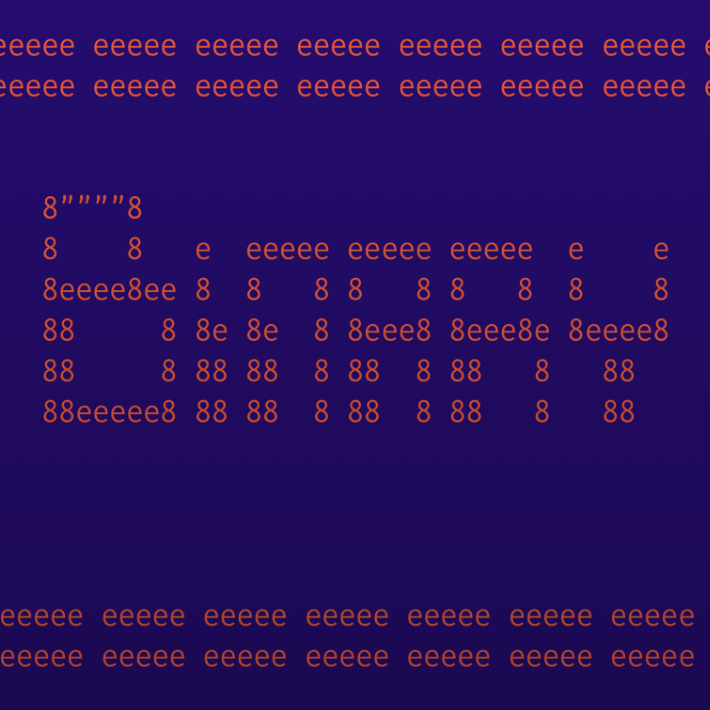
Genrenator API - Binary Jazz
MusicA Look at the Binary Jazz Genrenator API
The Binary Jazz Genrenator API is a free public API that generates random music genres on the fly. With this API, developers have access to over 350 different genres, allowing them to add excitement and exploration to their music-related projects.
Here are some examples of how to use the Binary Jazz Genrenator API in JavaScript:
Getting Started
To get started, you will want to include the following code at the top of your file. This will allow you to import the axios library, which is a required dependency for using this API.
const axios = require('axios');
Generating a Random Genre
To generate a random genre using the Binary Jazz Genrenator API, you can use the following code:
axios.get('https://binaryjazz.us/wp-json/genrenator/v1/genre/')
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.log(error);
});
This code will make a GET request to the Genrenator API and log the response to the console. The response will be a JSON object containing a single property called "genre" that holds the generated genre.
Getting Multiple Genres
To generate multiple genres at once, you can use a for loop to make multiple requests to the Genrenator API. For example:
for (let i = 0; i < 5; i++) {
axios.get('https://binaryjazz.us/wp-json/genrenator/v1/genre/')
.then(function (response) {
console.log(response.data.genre);
})
.catch(function (error) {
console.log(error);
});
}
This code will make five requests to the Genrenator API and log the generated genres to the console.
Conclusion
With the Binary Jazz Genrenator API, it's easy to add a bit of excitement and exploration to your music-related projects. By using these examples in JavaScript, you can quickly get started and begin generating random genres in no time.