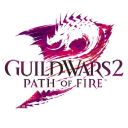
Guild Wars 2
Games & ComicsExploring the Guild Wars 2 API with JavaScript
The Guild Wars 2 API is a public API that allows developers to access information about the game and its playerbase. With the help of JavaScript, we can make API requests and parse the JSON data that is returned.
Getting Started
To begin using the API, we will need to create an API key. This can be done on the Guild Wars 2 account management page. Once we have our API key, we can begin making requests.
To make our requests in JavaScript, we will be using the Fetch API. This is a modern replacement for XMLHttpRequest
and allows us to make HTTP requests in a much simpler and cleaner way.
Example Requests
Here are some example requests using the Guild Wars 2 API and JavaScript:
Retrieve account information
const apiKey = '<your-api-key>';
fetch(`https://api.guildwars2.com/v2/account?access_token=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
This request will return information about the account associated with the provided API key.
Retrieve character information
const apiKey = '<your-api-key>';
const characterName = 'Character Name';
fetch(`https://api.guildwars2.com/v2/characters/${encodeURIComponent(characterName)}?access_token=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
This request will return information about the specified character. Be sure to URL encode the character name in the request URL.
Retrieve item information
const itemId = 12345;
fetch(`https://api.guildwars2.com/v2/items/${itemId}`)
.then(response => response.json())
.then(data => console.log(data));
This request will return information about the item with the specified ID.
Retrieve achievement information
const achievementId = 12345;
fetch(`https://api.guildwars2.com/v2/achievements/${achievementId}`)
.then(response => response.json())
.then(data => console.log(data));
This request will return information about the achievement with the specified ID.
Conclusion
The Guild Wars 2 API is a powerful tool for developers looking to build applications and tools around the game. With the help of JavaScript and the Fetch API, we can easily make API requests and retrieve data. Happy coding!