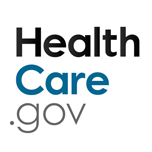
Healthcare.gov
HealthExploring Healthcare.gov API
If you are interested in healthcare data or you're building an application in the healthcare sector, then you are in the right place!
Healthcare.gov provides a set of APIs giving developers access to healthcare data (i.e., health plans available on the individual and small group markets), part of President Obama’s Affordable Care Act. In this blog, we will explore how to use the Healthcare.gov API in Javascript.
Getting Started
First, let's explore Healthcare.gov's API documentation. Follow the below steps to get started:
-
Visit the Healthcare.gov Developers Website at https://www.healthcare.gov/developers/. Here, you will find API documentation and information on how to register to use the API.
-
Once you have registered, you will receive an API key which can be used to make API requests.
JavaScript Examples
Here are a few examples of how to make API requests to Healthcare.gov using Javascript:
Example 1: Retrieving a Health Plan
const apiKey = 'your-api-key';
const planId = '123456';
fetch(`https://api.healthcare.gov/api/glossary/${planId}?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Example 2: Searching for Health Plans
const apiKey = 'your-api-key';
const state = 'DC';
const zipCode = '20002';
fetch(`https://api.healthcare.gov/v3.0/states/${state}/plans/search?zip=${zipCode}&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Example 3: Retrieving Health Plan Data by State and County
const apiKey = 'your-api-key';
const state = 'VA';
const county = '51059';
fetch(`https://www.healthcare.gov/api/glossary/region/state/${state}/county/${county}/?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
Healthcare.gov's API provides valuable healthcare data. In this blog, we explored how to use the API in Javascript with a few examples. We hope that you found this blog informative and that it helps you get started with using Healthcare.gov's API for your healthcare application. Happy coding!