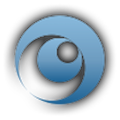
Hubblesite
NewsExploring the HubbleSite Public API
HubbleSite is a site that showcases images and data from the Hubble Space Telescope. They offer a public API that allows developers to access this data programmatically. In this blog post, we'll explore some of the endpoints available in the API and provide example code in JavaScript.
Getting Started
To use the HubbleSite API, you'll need an API key, which you can get by creating a free account on their website. Once you have your API key, you can start making requests to the API endpoints.
All responses from the API are returned as JSON objects.
API Endpoints
/images
This endpoint returns a list of all the images available on HubbleSite. You can filter the results by various parameters, such as image type, collection name, and object name.
const apiKey = YOUR_API_KEY;
const apiUrl = 'http://hubblesite.org/api/v3/images';
async function fetchImages() {
const response = await fetch(`${apiUrl}?page=all&api_key=${apiKey}`);
const data = await response.json();
return data;
}
fetchImages()
.then(data => console.log(data))
.catch(error => console.error(error));
/image/{id}
This endpoint retrieves the metadata for a specific image by its ID.
const apiKey = YOUR_API_KEY;
const apiUrl = 'http://hubblesite.org/api/v3/image';
async function fetchImage(id) {
const response = await fetch(`${apiUrl}/${id}?api_key=${apiKey}`);
const data = await response.json();
return data;
}
fetchImage(1)
.then(data => console.log(data))
.catch(error => console.error(error));
/news
This endpoint retrieves news articles from HubbleSite.
const apiKey = YOUR_API_KEY;
const apiUrl = 'http://hubblesite.org/api/v3/news';
async function fetchNews() {
const response = await fetch(`${apiUrl}?page=all&api_key=${apiKey}`);
const data = await response.json();
return data;
}
fetchNews()
.then(data => console.log(data))
.catch(error => console.error(error));
/news_release/{id}
This endpoint retrieves a specific news article by its ID.
const apiKey = YOUR_API_KEY;
const apiUrl = 'http://hubblesite.org/api/v3/news_release';
async function fetchNewsRelease(id) {
const response = await fetch(`${apiUrl}/${id}?api_key=${apiKey}`);
const data = await response.json();
return data;
}
fetchNewsRelease(1)
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
The HubbleSite API provides a wealth of information and images from the Hubble Space Telescope, allowing developers to create unique and engaging applications. In this post, we explored some of the available endpoints and provided example code in JavaScript. Happy coding!