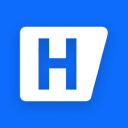
Human API
HealthAccessing Health Data Made Easy with Human API
Are you tired of manually collecting health data from different sources? Human API is here to make your life easier. With this public API, you can retrieve health data from hundreds of sources, including electronic health records, medical devices, fitness apps, and more.
Getting Started with Human API
To use Human API, you will need to sign up for a RapidAPI account and subscribe to the Human API endpoint. Once you have completed these steps, you will receive an API key that you can use to authenticate your requests.
Example Code in JavaScript
Here are some example code snippets in JavaScript that demonstrate how to use the Human API to retrieve health data:
Retrieve User Data
To retrieve a user's data, you can use the following API endpoint:
fetch("https://humanapi.p.rapidapi.com/v1/human", {
"method": "GET",
"headers": {
"x-rapidapi-host": "humanapi.p.rapidapi.com",
"x-rapidapi-key": "YOUR_API_KEY"
}
})
.then(response => {
console.log(response);
})
.catch(err => {
console.error(err);
});
Retrieve Activity Data
To retrieve a user's activity data, you can use the following API endpoint:
fetch("https://humanapi.p.rapidapi.com/v1/human/activities/daily_summary?date=2022-01-01", {
"method": "GET",
"headers": {
"x-rapidapi-host": "humanapi.p.rapidapi.com",
"x-rapidapi-key": "YOUR_API_KEY"
}
})
.then(response => {
console.log(response);
})
.catch(err => {
console.error(err);
});
Retrieve Heart Rate Data
To retrieve a user's heart rate data, you can use the following API endpoint:
fetch("https://humanapi.p.rapidapi.com/v1/human/heart_rate?start_time=2022-01-01T00:00:00Z&end_time=2022-01-02T00:00:00Z", {
"method": "GET",
"headers": {
"x-rapidapi-host": "humanapi.p.rapidapi.com",
"x-rapidapi-key": "YOUR_API_KEY"
}
})
.then(response => {
console.log(response);
})
.catch(err => {
console.error(err);
});
Retrieve Sleep Data
To retrieve a user's sleep data, you can use the following API endpoint:
fetch("https://humanapi.p.rapidapi.com/v1/human/sleeps?start_time=2022-01-01T00:00:00Z&end_time=2022-01-02T00:00:00Z", {
"method": "GET",
"headers": {
"x-rapidapi-host": "humanapi.p.rapidapi.com",
"x-rapidapi-key": "YOUR_API_KEY"
}
})
.then(response => {
console.log(response);
})
.catch(err => {
console.error(err);
});
Conclusion
Human API makes it easy to collect health data from different sources. With its extensive range of endpoints, you can retrieve data such as activity, heart rate, and sleep information. By using the example code snippets provided in this post, you can start integrating the Human API into your application today!