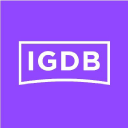
IGDB.com
Games & ComicsIntroduction to IGDB API
The IGDB API is a public API which provides information on video games, ranging from game release dates to character and developer information. This API can be used to extract data for building various gaming related web and mobile applications. In this blog post, we will explore the IGDB API and provide examples of how to use it using JavaScript.
Getting Started
To get started with the IGDB API, you will need to create an account on https://api.igdb.com/ and obtain an access token. The access token can be generated by going to the API section of the dashboard on the website and creating an app. Once you have the access token, you can start making API requests.
Example API Request
To make an API request, you need to use the Fetch API provided by JavaScript. The below JavaScript code will demonstrate how to make a simple request to get information about a video game:
const apiKey = 'YOUR_API_KEY';
const endpoint = 'https://api.igdb.com/v4/games';
const body = 'fields name,release_dates.*,cover.*,genres.*; where name = "Halo 5: Guardians";';
const headers = new Headers();
headers.append('Accept', 'application/json');
headers.append('Client-ID', apiKey);
headers.append('Authorization', `Bearer ${accessToken}`);
fetch(endpoint, {
method: 'POST',
headers,
body
}).then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we first set our API key and endpoint URL. The body
variable contains the query we want to run against the API. It includes the game name we want to retrieve, as well as the fields we want to retrieve about that game.
We then set the headers required for the API request. These include the Accept
header, which specifies the response format, the Client-ID
header, which specifies our client ID, and the Authorization
header, which specifies our access token.
Finally, we use the fetch API to make a POST request to the endpoint with the provided headers and body. We then parse the JSON response and log it to the console.
Conclusion
In this blog post, we have provided an introduction to the IGDB API and demonstrated how to make a simple request using JavaScript. The IGDB API provides a wealth of information on video games that can be used to build various gaming related applications.