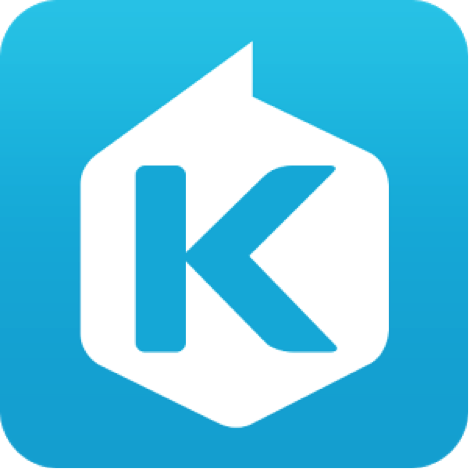
KKBOX
MusicHow to Use the KKBOX Public API
KKBOX provides a public API that developers can use to access and retrieve data from their music streaming service. In this guide, we will be going through the steps needed to get started, as well as provide examples of how to use the API in JavaScript.
Step 1: Register for an Account and Get Your API Key
Before you can start using the KKBOX API, you will need to create an account on the KKBOX Developer Site. Once you have created an account, you can generate your unique API key that you will need to use every time you make a request to the API.
Step 2: Familiarize Yourself with the API Documentation
The next step is to familiarize yourself with the KKBOX API documentation. This documentation provides a detailed explanation of the available endpoints, parameters, and response formats. You can find the documentation at the following link: https://docs-en.kkbox.codes/reference
Step 3: Example API Codes in JavaScript
Example 1: Get Top Tracks by Country
This example gets the top tracks by country from the KKBOX API. Replace your_client_id
and your_client_secret
with your own credentials.
fetch('https://account.kkbox.com/oauth2/token', {
method: 'POST',
body: 'grant_type=client_credentials&client_id=your_client_id&client_secret=your_client_secret',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
})
.then(response => response.json())
.then(data => {
fetch(`https://api.kkbox.com/v1.1/charts?territory=TW&category=track&offset=0&limit=10`, {
headers: {
'Authorization': `Bearer ${data.access_token}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.log(err));
})
.catch(err => console.log(err));
Example 2: Search Tracks by Keyword
This example searches tracks by keyword from the KKBOX API. Replace your_client_id
and your_client_secret
with your own credentials.
fetch('https://account.kkbox.com/oauth2/token', {
method: 'POST',
body: 'grant_type=client_credentials&client_id=your_client_id&client_secret=your_client_secret',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
})
.then(response => response.json())
.then(data => {
fetch(`https://api.kkbox.com/v1.1/search?q=${encodeURIComponent('Jay Chou')}&type=track&offset=0&limit=10`, {
headers: {
'Authorization': `Bearer ${data.access_token}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.log(err));
})
.catch(err => console.log(err));
Conclusion
Using the KKBOX API in JavaScript is a great way to access and retrieve data from their music streaming service. By following the steps outlined in this guide, you should be able to successfully access and retrieve data from the API using JavaScript.