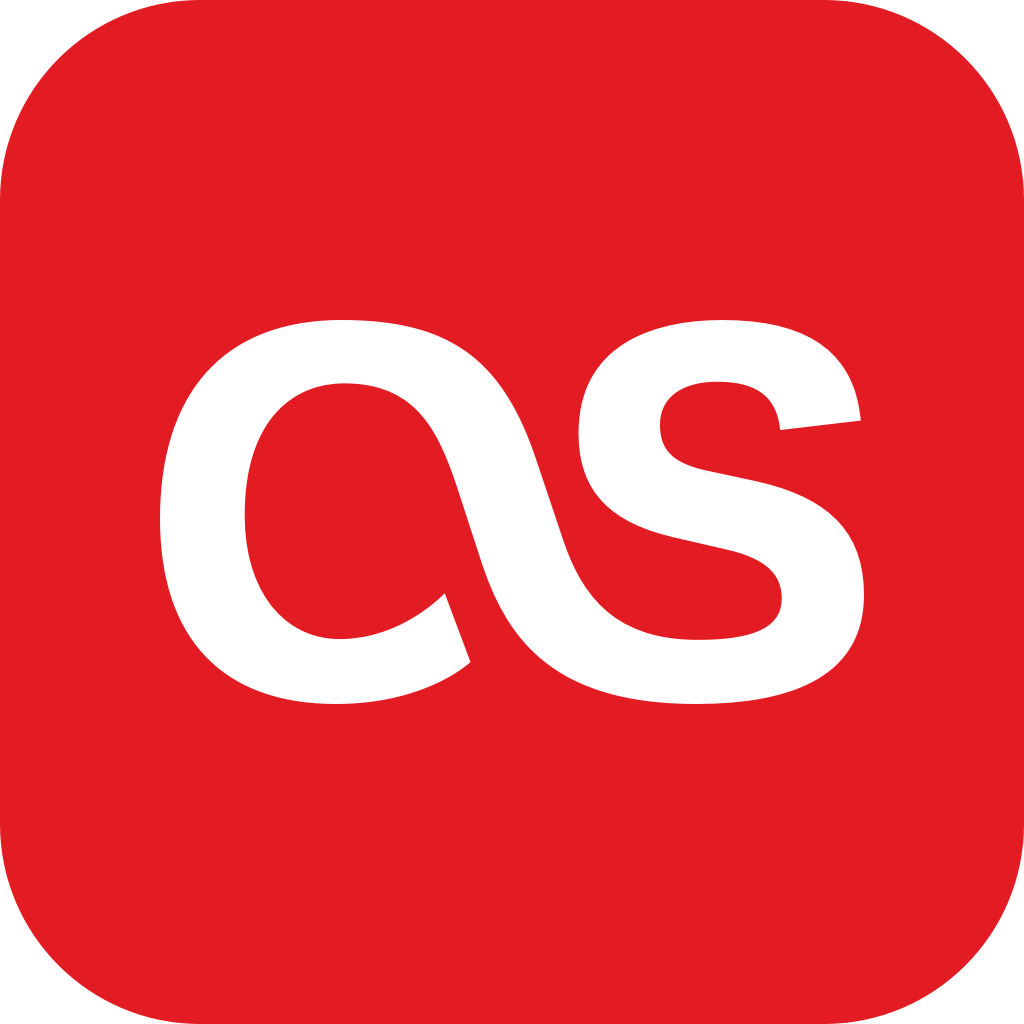
LastFm
MusicUsing Last.fm API in JavaScript
Last.fm provides a public API that allows developers to access information about music, artists, tags, and more. In this blog post, we'll explore some of the basic API requests using JavaScript.
Getting Started
Before we can make API requests, we need to obtain an API key from Last.fm. We can do this by creating an account on the Last.fm website and then applying for an API key. Once we have the API key, we can start making requests.
Making API Requests
Last.fm API requests can be made using any programming language that can communicate with web APIs. In this example, we'll use JavaScript to make API requests using the fetch
method.
Search for Songs
To search for songs, we can use the track.search
method. Here's an example code snippet:
const apiKey = '<your-API-key>';
const searchText = 'love';
fetch(`http://ws.audioscrobbler.com/2.0/?method=track.search&track=${searchText}&api_key=${apiKey}&format=json`)
.then(response => response.json())
.then(data => console.log(data));
In this code, we construct the API URL by building a query string with the API method (track.search
), the search text (love
), our API key, and the response format (json
). We then use fetch
to make a GET request to the Last.fm API with this URL.
Get Song Info
To get information about a specific song, we can use the track.getInfo
method. Here's an example code snippet:
const apiKey = '<your-API-key>';
const trackArtist = 'The Beatles';
const trackName = 'Let It Be';
fetch(`http://ws.audioscrobbler.com/2.0/?method=track.getInfo&api_key=${apiKey}&artist=${trackArtist}&track=${trackName}&format=json`)
.then(response => response.json())
.then(data => console.log(data));
In this code, we construct the API URL by building a query string with the API method (track.getInfo
), our API key, the song artist (The Beatles
), the song name (Let It Be
), and the response format (json
). We then use fetch
to make a GET request to the Last.fm API with this URL.
Get Artist Info
To get information about a specific artist, we can use the artist.getInfo
method. Here's an example code snippet:
const apiKey = '<your-API-key>';
const artistName = 'The Beatles';
fetch(`http://ws.audioscrobbler.com/2.0/?method=artist.getInfo&api_key=${apiKey}&artist=${artistName}&format=json`)
.then(response => response.json())
.then(data => console.log(data));
In this code, we construct the API URL by building a query string with the API method (artist.getInfo
), our API key, the artist name (The Beatles
), and the response format (json
). We then use fetch
to make a GET request to the Last.fm API with this URL.
Conclusion
Using the Last.fm API in JavaScript is a great way to access information about music and artists. By constructing API URLs and making requests using fetch
, we can easily retrieve data and integrate it into our own applications. This was just a brief introduction to some of the basic API requests, but the Last.fm API offers many more methods and parameters to explore.