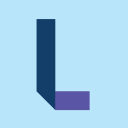
Lexigram
HealthExploring the Lexigram API with JavaScript
The Lexigram API is a powerful tool for working with healthcare data. It provides access to a wide range of medical and clinical data, allowing developers to build innovative solutions that can help improve patient care.
In this blog post, we will explore the Lexigram API with JavaScript. We'll look at some sample code that demonstrates how to interact with the API and extract useful information from healthcare documents.
Getting Started
To get started with the Lexigram API, you will need an API key. You can get one by following the instructions on the Lexigram API website.
Once you have your API key, you can start exploring the API. To keep things simple, we'll focus on a few of the most commonly used endpoints.
Entity Extraction
One of the most powerful features of the Lexigram API is its entity extraction functionality. Entity extraction allows you to extract useful information from healthcare documents, such as the names of drugs, treatments, and diseases mentioned in the text.
To use the entity extraction endpoint, simply send a POST request to the following URL:
https://api.lexigram.io/v1/extract
Here's an example of how to call this endpoint from JavaScript:
const apiKey = 'YOUR_API_KEY_HERE';
const text = 'The patient is currently taking aspirin for high blood pressure.';
fetch('https://api.lexigram.io/v1/extract', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + apiKey
},
body: JSON.stringify({
text: text,
target: 'all'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're sending a POST request to the /extract
endpoint with a simple text string. The target
parameter specifies which types of entities we want to extract (in this case, we're extracting all types of entities).
The response will be a JSON object containing information about the entities that were extracted from the text. Here's an example response:
{
"success": true,
"data": [
{
"type": "drug",
"name": "aspirin",
"source": "RxNorm"
},
{
"type": "condition",
"name": "high blood pressure",
"source": "SNOMED CT"
}
]
}
As you can see, the response includes the type of each entity, its name, and the source of the information (in this case, the source is either RxNorm or SNOMED CT).
Similar Entities
Another useful endpoint in the Lexigram API is the similar entities endpoint. This endpoint allows you to find entities that are similar to a given entity based on their text and context.
To use the similar entities endpoint, send a GET request to the following URL:
https://api.lexigram.io/v1/similar_entities?text={text}
Replace {text}
with the entity you want to find similar entities for.
Here's an example of how to call this endpoint from JavaScript:
const apiKey = 'YOUR_API_KEY_HERE';
const entityText = 'aspirin';
fetch(`https://api.lexigram.io/v1/similar_entities?text=${entityText}`, {
method: 'GET',
headers: {
'Authorization': 'Bearer ' + apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're sending a GET request to the /similar_entities
endpoint with the text
parameter set to 'aspirin'
. The response will be a JSON object containing information about entities that are similar to aspirin.
Search
Finally, the Lexigram API also provides a search endpoint that allows you to search its database of healthcare information. This endpoint can be used to find information about specific drugs, treatments, or conditions, as well as to search for articles or other types of healthcare content.
To use the search endpoint, send a GET request to the following URL:
https://api.lexigram.io/v1/search?q={query}
Replace {query}
with the search term you want to use.
Here's an example of how to call this endpoint from JavaScript:
const apiKey = 'YOUR_API_KEY_HERE';
const query = 'aspirin';
fetch(`https://api.lexigram.io/v1/search?q=${query}`, {
method: 'GET',
headers: {
'Authorization': 'Bearer ' + apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're sending a GET request to the /search
endpoint with the q
parameter set to 'aspirin'
. The response will be a JSON object containing information about search results that match the query.
Conclusion
In this blog post, we've seen how to use the Lexigram API with JavaScript. We explored three of the most commonly used endpoints: entity extraction, similar entities, and search. By leveraging the power of the Lexigram API, you can build innovative healthcare applications that can help improve patient care and outcomes.