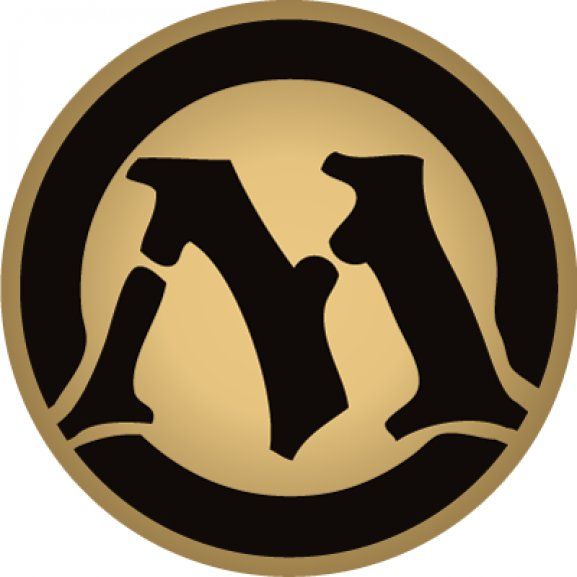
Magic the gathering
Games & ComicsExplore the Magic: The Gathering API with JavaScript
Magic: The Gathering is a popular trading card game with an extensive set of characters, spells, and creatures. To create applications that interact with the game's data, Magic: The Gathering provides a public API that returns information on cards, sets, and other game elements. In this blog post, we will explore how to use the Magic: The Gathering API with JavaScript.
Getting Started
To get started, we will need to request an API key from Magic: The Gathering. You can obtain your API key by registering for free at http://magicthegathering.io/. Once you have your API key, we can proceed with creating a JavaScript application that accesses and processes the data exposed by the API.
API Endpoints
The Magic: The Gathering API provides several endpoints that return different types of information. Here are some examples:
/cards
This endpoint returns information about a specific Magic: The Gathering card, specified by its multiverse ID, name, or set.
Example Usage
fetch('https://api.magicthegathering.io/v1/cards?name=Bloodfire')
.then(response => response.json())
.then(data => console.log(data));
/sets
This endpoint returns information about a specific Magic: The Gathering set, specified by its code or name.
Example Usage
fetch('https://api.magicthegathering.io/v1/sets/ORI')
.then(response => response.json())
.then(data => console.log(data));
/types
This endpoint returns a list of all Magic: The Gathering card types.
Example Usage
fetch('https://api.magicthegathering.io/v1/types')
.then(response => response.json())
.then(data => console.log(data));
API Query Parameters
The Magic: The Gathering API also allows us to filter and sort the returned data by using query parameters. Here are some examples:
Filter by Set
fetch('https://api.magicthegathering.io/v1/cards?set=ORI')
.then(response => response.json())
.then(data => console.log(data));
Filter by Rarity
fetch('https://api.magicthegathering.io/v1/cards?rarity=Rare')
.then(response => response.json())
.then(data => console.log(data));
Sort by Power
fetch('https://api.magicthegathering.io/v1/cards?orderBy=power')
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In this blog post, we explored the Magic: The Gathering API and learned how to access and process the data it returns using JavaScript. We also looked at some of the API query parameters that allow us to filter and sort the returned data. With this knowledge, you can now create exciting applications that interact with the Magic: The Gathering game data!