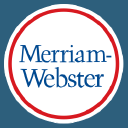
Merriam-Webster
DictionariesUsing Dictionary API with JavaScript
Dictionary API is a great source of information for developers looking to add dictionary and thesaurus functionality to their app. With this API, you can easily fetch definitions, synonyms, antonyms and more. In this article, we will explore how to use the Dictionary API with JavaScript.
Getting Started with Dictionary API
Before you can start using the Dictionary API, you will need to sign up for an API key. You can sign up for free at dictionaryapi.com. Once you have an API key, you can start making API requests.
Fetching Definitions
To fetch a definition from the Dictionary API, you will need to make a GET request to the API endpoint with the word you want to search. Here's an example code snippet on how to fetch a definition using JavaScript:
const API_KEY = 'YOUR_API_KEY';
const WORD = 'test';
fetch(`https://www.dictionaryapi.com/api/v3/references/collegiate/json/${WORD}?key=${API_KEY}`)
.then(response => response.json())
.then(data => {
const definition = data[0].shortdef[0];
console.log(definition);
})
.catch(error => console.log(error));
This code sample shows how to fetch the definition of a word using the fetch API. Replace the API_KEY
variable with your API key, and WORD
variable with the word you want to search.
Fetching Synonyms
To fetch synonyms for a word, make a GET request to the API endpoint with the synonyms
parameter. Here's an example code snippet on how to fetch synonyms using JavaScript:
const API_KEY = 'YOUR_API_KEY';
const WORD = 'test';
fetch(`https://www.dictionaryapi.com/api/v3/references/collegiate/json/${WORD}?key=${API_KEY}&synonyms`)
.then(response => response.json())
.then(data => {
const synonyms = data[0].meta.syns[0].join(', ');
console.log(`Synonyms: ${synonyms}`);
})
.catch(error => console.log(error));
This code sample shows how to fetch synonyms of a word using the Dictionary API. Replace the API_KEY
variable with your API key, and WORD
variable with the word you want to search.
Fetching Antonyms
To fetch antonyms for a word, make a GET request to the API endpoint with the antonyms
parameter. Here's an example code snippet on how to fetch antonyms using JavaScript:
const API_KEY = 'YOUR_API_KEY';
const WORD = 'test';
fetch(`https://www.dictionaryapi.com/api/v3/references/collegiate/json/${WORD}?key=${API_KEY}&antonyms`)
.then(response => response.json())
.then(data => {
const antonyms = data[0].meta.ants[0].join(', ');
console.log(`Antonyms: ${antonyms}`);
})
.catch(error => console.log(error));
This code sample shows how to fetch antonyms of a word using the Dictionary API. Replace the API_KEY
variable with your API key, and WORD
variable with the word you want to search.
Fetching Related Words
To fetch related words for a word, make a GET request to the API endpoint with the rel_jja
or rel_jjb
parameter. Here's an example code snippet on how to fetch related words using JavaScript:
const API_KEY = 'YOUR_API_KEY';
const WORD = 'test';
fetch(`https://www.dictionaryapi.com/api/v3/references/collegiate/json/${WORD}?key=${API_KEY}&rel_jja`)
.then(response => response.json())
.then(data => {
const relatedWords = data[0].meta.jja[0].join(', ');
console.log(`Related Words: ${relatedWords}`);
})
.catch(error => console.log(error));
This code sample shows how to fetch related words of a word using the Dictionary API. Replace the API_KEY
variable with your API key, and WORD
variable with the word you want to search.
Conclusion
Using the Dictionary API with JavaScript is simple. Whether you want to fetch definitions, synonyms, antonyms, or related words, the Dictionary API provides a reliable source of information for all your dictionary needs. Happy coding!