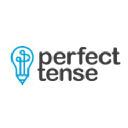
Perfect Tense
DictionariesThe Perfect Tense API is an artificial intelligence powered spelling and grammar API. All you have to do is provide a piece of text and the Perfect Tense API will automatically return a proofread version of the text, along with all spelling and grammar mistakes.
📚 Documentation & Examples
Everything you need to integrate with Perfect Tense
🚀 Quick Start Examples
// Perfect Tense API Example
const response = await fetch('https://www.perfecttense.com/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Understanding the Perfect Tense Public API
Perfect Tense is an online tool that helps you check the grammar and spelling of your content. The Perfect Tense API allows developers to incorporate this functionality into their own apps, websites, and platforms. In this short blog post, we will explore the Perfect Tense Public API and provide some example code in JavaScript.
Getting Started
To get started, you will need to sign up for a Perfect Tense API Key. Once you have your API key, you can use it to authenticate requests to the API. The Perfect Tense API uses a RESTful API architecture, which means that you can use HTTP requests to get data from and send data to the API.
Example Code
Let's take a look at some example code in JavaScript that demonstrates how to use the Perfect Tense API.
Spell Check
This example code demonstrates how to use the Perfect Tense API to perform a spell check on a piece of text.
const apiKey = 'YOUR_API_KEY';
const text = 'The quick brown fox jump over the lazy dog.';
const url = `https://api.perfecttense.com/v3/spelling?text=${encodeURIComponent(text)}&api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Grammar Check
This example code demonstrates how to use the Perfect Tense API to perform a grammar check on a piece of text.
const apiKey = 'YOUR_API_KEY';
const text = 'The quick brown fox jump over the lazy dog.';
const url = `https://api.perfecttense.com/v3/grammar?text=${encodeURIComponent(text)}&api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Proofreading
This example code demonstrates how to use the Perfect Tense API to perform a proofreading check on a piece of text.
const apiKey = 'YOUR_API_KEY';
const text = 'The quick brown fox jump over the lazy dog.';
const url = `https://api.perfecttense.com/v3/proofreading?text=${encodeURIComponent(text)}&api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In this blog post, we have explored the Perfect Tense Public API and provided some example code in JavaScript. These examples demonstrate how to use the Perfect Tense API to perform spelling, grammar, and proofreading checks on your content. With the Perfect Tense API, you can easily integrate this functionality into your own applications and platforms, giving your users access to powerful proofreading tools.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes