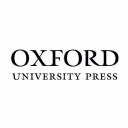
Oxford
DictionariesUsing Oxford Dictionaries API in JavaScript
If you're looking to incorporate Oxford Dictionaries' API into your JavaScript app, you're in luck! The API is available for free and you can get started right away.
First, let's set up our app to make requests to the API. We'll be using axios
for this example. You can install it using npm:
npm install axios
Now let's import it in our JavaScript file:
import axios from "axios";
Getting an API Key
Before we can use the Oxford Dictionaries API, we need to get an API key. You can sign up for a free account here.
After registering, you can create a new project and get your app_id
and app_key
.
Making API Calls
Now we're ready to make requests to the API.
Getting the Definition of a Word
Let's start with the simplest example: getting the definition of a word. We'll use the /entries
endpoint for this.
const getDefinition = async (word) => {
const url = `https://od-api.oxforddictionaries.com/api/v2/entries/en-us/${word}`;
const headers = {
app_id: YOUR_APP_ID,
app_key: YOUR_APP_KEY
};
const result = await axios.get(url, { headers });
const definition = result.data.results[0].lexicalEntries[0].entries[0].senses[0].definitions[0];
return definition;
}
You'll need to replace YOUR_APP_ID
and YOUR_APP_KEY
with your own credentials.
Now you can call the getDefinition
function with a word and it will return a Promise that resolves to the definition.
getDefinition("hello").then((definition) => console.log(definition));
// Output: used as a greeting or to begin a telephone conversation
Getting Synonyms of a Word
Next, let's find some synonyms for a word. We'll use the /thesaurus
endpoint for this.
const getSynonyms = async (word) => {
const url = `https://od-api.oxforddictionaries.com/api/v2/thesaurus/en/${word}`;
const headers = {
app_id: YOUR_APP_ID,
app_key: YOUR_APP_KEY
};
const result = await axios.get(url, { headers });
const synonyms = result.data.results[0].lexicalEntries[0].entries[0].senses[0].synonyms;
return synonyms;
}
Again, replace YOUR_APP_ID
and YOUR_APP_KEY
with your own credentials.
Now you can call the getSynonyms
function with a word and it will return a Promise that resolves to an array of synonyms.
getSynonyms("happy").then((synonyms) => console.log(synonyms));
// Output: [ 'content', 'pleased', 'delighted', ... ]
Getting Example Sentences of a Word
Finally, let's get some example sentences using a word. We'll use the /sentences
endpoint for this.
const getExampleSentences = async (word) => {
const url = `https://od-api.oxforddictionaries.com/api/v2/sentences/en-us/${word}`;
const headers = {
app_id: YOUR_APP_ID,
app_key: YOUR_APP_KEY
};
const result = await axios.get(url, { headers });
const sentences = result.data.results[0].lexicalEntries[0].sentences.map((sentence) => sentence.text);
return sentences;
}
Again, replace YOUR_APP_ID
and YOUR_APP_KEY
with your own credentials.
Now you can call the getExampleSentences
function with a word and it will return a Promise that resolves to an array of example sentences.
getExampleSentences("world").then((sentences) => console.log(sentences));
// Output: [ 'the countries of the world', 'The world is a beautiful place to live.' ]
Conclusion
That's all there is to it! Now you can use the Oxford Dictionaries API to add rich language features to your JavaScript app.