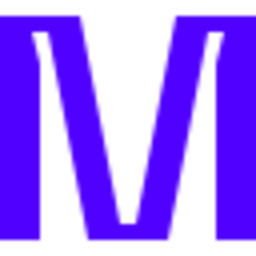
Mixcloud
MusicUsing the Mixcloud API with JavaScript
The Mixcloud API allows developers to access data from the popular audio streaming platform. In this blog post, we'll explore how to use the Mixcloud API with JavaScript, using various API examples.
Authentication
Before you can make any API requests, you'll need to register a new application on the Mixcloud Developers website. Once you've created a new application, you'll be provided with a client_id
and client_secret
which you'll use to authenticate your API requests.
const client_id = 'YOUR_CLIENT_ID';
const client_secret = 'YOUR_CLIENT_SECRET';
const access_token = 'YOUR_ACCESS_TOKEN';
User API
The User API provides information about Mixcloud users, such as their username, follower count, and uploads. Here's an example of how to retrieve details about a specific user using their username:
const username = 'EXAMPLE_USER';
const userUrl = `https://api.mixcloud.com/${username}/`;
fetch(userUrl)
.then(response => response.json())
.then(data => console.log(data));
Track API
The Track API provides information about individual audio tracks on Mixcloud. Here's an example of how to retrieve details about a specific track using its URL:
const trackUrl = 'https://www.mixcloud.com/mixes/cloudcasts/EXAMPLE_TRACK/';
const apiUrl = `${trackUrl}?metadata=1`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
Search API
The Search API allows you to find tracks, users, and other items on Mixcloud. Here's an example of how to search for tracks by a specific user:
const username = 'EXAMPLE_USER';
const query = 'EXAMPLE_QUERY';
const searchUrl = `https://api.mixcloud.com/search/?q=${query}&type=cloudcast&user=${username}`;
fetch(searchUrl)
.then(response => response.json())
.then(data => console.log(data));
Uploader API
The Uploader API allows you to create, edit and delete audio tracks on Mixcloud. Here's an example of how to upload a new track using the API:
const uploadUrl = 'https://api.mixcloud.com/upload/';
fetch(uploadUrl, {
method: 'POST',
headers: {
'Authorization': `Bearer ${access_token}`,
'Content-Type': 'multipart/form-data'
},
body: formData
})
.then(response => response.json())
.then(data => console.log(data));
In conclusion, the Mixcloud API is a powerful tool that allows developers to tap into the platform's vast audio library and user base. With the examples above, you should be able to get started using the Mixcloud API with JavaScript.