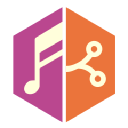
MusicBrainz
MusicMusicBrainz Public API Docs
MusicBrainz is a community-maintained open-source database that collects information about music artists, releases, and tracks. It provides a public API that allows developers to access this rich data and build innovative music applications.
The MusicBrainz Public API supports REST conventions over HTTP through XML web services. This documentation covers the Version 2 of the MusicBrainz API. In this blog, we will explore the MusicBrainz API and how to use it with JavaScript.
Getting Started
To get started with the MusicBrainz API, you need to create an account on the MusicBrainz website. With your account, you can generate an API key that you will need to include in the headers of your requests.
Let's walk through an example of how to use the MusicBrainz API and retrieve information about an artist using JavaScript:
const artistName = 'Coldplay';
const requestUrl = `https://musicbrainz.org/ws/2/artist?query=${artistName}&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.artists[0].name);
})
.catch((error) => {
console.error(error);
});
In this example, we are making a GET request to the MusicBrainz API to search for an artist named Coldplay. We are also including our API key in the Authorization
header of the request.
Once we receive the response from the API, we extract the name of the first artist that matches the search query and log it to the console.
API Endpoints
The MusicBrainz API provides endpoints to retrieve different types of data including artists, releases, and tracks. Here are some of the endpoints that you can use with JavaScript:
Search Artists
const artistName = 'Linkin Park';
const requestUrl = `https://musicbrainz.org/ws/2/artist?query=${artistName}&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.artists);
})
.catch((error) => {
console.error(error);
});
Get Artist
const artistId = '0039c7ae-e1a7-4a7d-9b49-0cbc716821a6';
const requestUrl = `https://musicbrainz.org/ws/2/artist/${artistId}?inc=aliases&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.name);
})
.catch((error) => {
console.error(error);
});
Search Releases
const artistName = 'Radiohead';
const requestUrl = `https://musicbrainz.org/ws/2/release?query=artist:${artistName}&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.releases);
})
.catch((error) => {
console.error(error);
});
Get Release
const releaseId = 'c032e638-1bea-4f0e-8e92-38182c3f560c';
const requestUrl = `https://musicbrainz.org/ws/2/release/${releaseId}?inc=labels&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.title);
})
.catch((error) => {
console.error(error);
});
Search Recordings
const trackName = 'Paradise';
const artistName = 'Coldplay';
const requestUrl = `https://musicbrainz.org/ws/2/recording?query=${trackName} AND artist:${artistName}&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.recordings);
})
.catch((error) => {
console.error(error);
});
Get Recording
const recordingId = 'ab197732-20e5-4e4b-8f20-02d5b589defb';
const requestUrl = `https://musicbrainz.org/ws/2/recording/${recordingId}?inc=isrcs&fmt=json`;
fetch(requestUrl, {
headers: {
'User-Agent': 'My Music App',
'Authorization': 'Bearer YOUR_API_KEY',
},
})
.then((response) => response.json())
.then((data) => {
console.log(data.title);
})
.catch((error) => {
console.error(error);
});
Conclusion
The MusicBrainz Public API provides a wealth of data that can be used to build innovative music applications. With the help of JavaScript, you can easily incorporate this data into your web application and create a seamless music browsing experience for your users.
Explore the MusicBrainz API documentation to discover more endpoints and start building amazing music apps today!