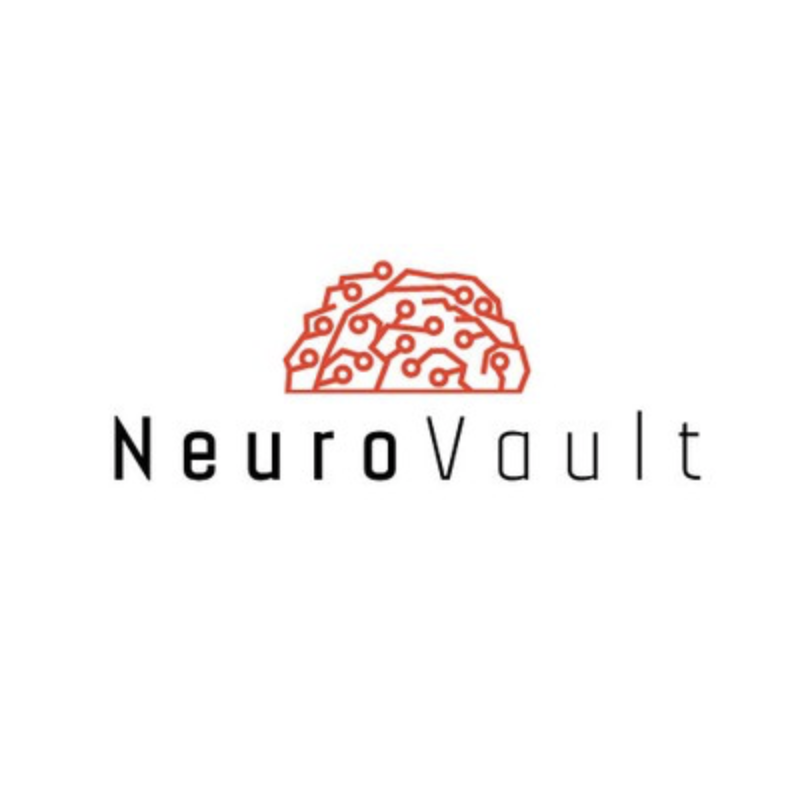
NeuroVault API
HealthIntroduction to NeuroVault API
NeuroVault is a database of brain imaging data that can be accessed through a public API. The API allows users to programmatically search and retrieve datasets using various parameters. In this blog post, we will explore the various API endpoints and provide examples of how to use them in JavaScript.
Installation
To use the NeuroVault API, you will need to install the axios
library. You can install it using npm
:
npm install axios
Retrieving datasets
To retrieve datasets from NeuroVault, you can use the /api/images
endpoint. The endpoint accepts several query parameters, including collection
, modality
, and map_type
. Here's an example of how to use the endpoint to retrieve all fMRI datasets in the 'brainomics' collection:
const axios = require('axios');
axios.get('https://neurovault.org/api/images', {
params: {
modality: 'fMRI',
collection: 'brainomics'
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Retrieving a single dataset
To retrieve a single dataset from NeuroVault, you can use the /api/images/<image_id>
endpoint. Here's an example of how to retrieve the dataset with the id 4567
:
const axios = require('axios');
axios.get('https://neurovault.org/api/images/4567')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Retrieving uploaded images
To retrieve the images uploaded by a specific user, you can use the /api/images/user/<username>
endpoint. Here's an example of how to retrieve all images uploaded by the user 'john':
const axios = require('axios');
axios.get('https://neurovault.org/api/images/user/john')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Retrieving collections
To retrieve collections from NeuroVault, you can use the /api/collections
endpoint. The endpoint accepts several query parameters, including owner
, public
, and name
. Here's an example of how to retrieve all public collections:
const axios = require('axios');
axios.get('https://neurovault.org/api/collections', {
params: {
public: 'true'
}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Retrieving a single collection
To retrieve a single collection from NeuroVault, you can use the /api/collections/<collection_id>
endpoint. Here's an example of how to retrieve the collection with id 1234
:
const axios = require('axios');
axios.get('https://neurovault.org/api/collections/1234')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Conclusion
In this blog post, we explored the various endpoints available in the NeuroVault API and provided examples of how to use them in JavaScript. The API provides a powerful tool for searching and retrieving brain imaging data, and can be integrated into a wide variety of applications.