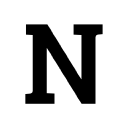
News API
NewsUsing the NewsAPI to Fetch News Articles in Your JavaScript Application
The NewsAPI is a popular and widely used public API that provides a simple interface for accessing the latest news articles from various sources. Whether you are building a web application, a mobile app, or a desktop application, the NewsAPI can help you integrate the latest news articles into your application with minimal effort.
In this blog post, we will explore how to use the NewsAPI to fetch news articles in your JavaScript application. We will cover the essential steps required to authenticate, send requests to the NewsAPI, and display the results. All of the code examples in this post will be written in JavaScript.
Prerequisites
Before we start, you will need to get an API key from the NewsAPI website. Head over to https://newsapi.org/register to sign up for a free API key.
Once you have your API key, you can start sending requests to the NewsAPI.
Sending a Request to the NewsAPI
The first step is to send a request to the NewsAPI to fetch the latest news articles. We will be using the fetch()
method to send requests to the NewsAPI, which is available in all modern browsers.
fetch(`https://newsapi.org/v2/top-headlines?country=us&apiKey=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
In the code example above, we are using the fetch()
method to send a GET request to the NewsAPI. We are passing in our API key as a query parameter, which is required to authenticate the request.
The NewsAPI provides various query parameters that you can use to filter the news articles based on criteria such as source, category, language, and more. In this example, we are using the country
parameter to fetch news articles from the United States.
The .then()
method is used to handle the response from the NewsAPI. We are parsing the response as JSON and logging it to the console.
The .catch()
method is used to handle any errors that may occur during the request.
Displaying the Results
Now that we have successfully sent a request to the NewsAPI and received a response, we can display the news articles in our application.
const newsContainer = document.querySelector('#news-container');
data.articles.forEach(article => {
const newsItem = document.createElement('div');
newsContainer.appendChild(newsItem);
const newsTitle = document.createElement('h2');
newsTitle.innerText = article.title;
newsItem.appendChild(newsTitle);
const newsDescription = document.createElement('p');
newsDescription.innerText = article.description;
newsItem.appendChild(newsDescription);
const newsImage = document.createElement('img');
newsImage.setAttribute('src', article.urlToImage);
newsItem.appendChild(newsImage);
const newsLink = document.createElement('a');
newsLink.setAttribute('href', article.url);
newsLink.innerText = 'Read More';
newsItem.appendChild(newsLink);
});
In the code example above, we are using the forEach()
method to iterate over the array of articles
in the response data. For each article, we are creating a new div
element to hold the article content.
We create a h2
element for the article title, a p
element for the article description, an img
element for the article image, and an a
element for the article link. We set the appropriate attributes and values for each element based on the data we received from the NewsAPI.
Finally, we append each element to the div
container using the appendChild()
method.
Conclusion
In this post, we explored how to use the NewsAPI to fetch news articles in your JavaScript application. We covered the essential steps required to authenticate, send requests to the NewsAPI, and display the results.
The NewsAPI provides an extensive API documentation that you can refer to for additional query parameters and endpoints. Head over to https://newsapi.org/docs to learn more.