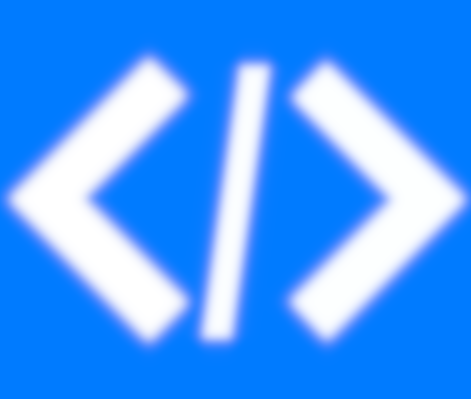
OkSurf
NewsExploring the Public API Docs of OK.Surf
Are you looking to integrate with OK.Surf's public API to access their data? Look no further! In this blog post, we’ll explore the OK.Surf API documentation and provide code examples written in JavaScript.
Getting Started
Before we start using the API, we’ll need to familiarize ourselves with the API documentation. The documentation is available at https://ok.surf/docs/api.
With that said, let’s get started with our first example.
Example 1: Retrieving Company Data
One of the most common use cases for an API is to retrieve data. To retrieve company data from OK.Surf, we can use the following JavaScript code:
fetch('https://api.ok.surf/companies')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code uses the fetch
function to make an HTTP GET request to the /companies
endpoint. The response is then parsed as JSON and logged to the console.
Example 2: Filtering Company Data
The OK.Surf API supports querying and filtering, allowing you to retrieve a specific subset of data. For example, to get all companies with the category
value set to "Tech", we can use the following code:
fetch('https://api.ok.surf/companies?filter[category]=Tech')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this code, we add a filter
parameter to the URL with the value of category=Tech
. This will filter the results to only include companies that have a category
value of "Tech".
Example 3: Adding a New Company
Now that we’ve seen how to retrieve data from the OK.Surf API, let's take a look at how to add new data. Here’s how to create a new company:
const formData = new FormData();
formData.append('name', 'My New Company');
formData.append('category', 'Tech');
fetch('https://api.ok.surf/companies', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we’re submitting a new company with the name "My New Company"
and the category "Tech"
. The API endpoint for creating a company is /companies
, which we pass along as the URL in our call to fetch
. We also provide the form data in the body
of the request as a FormData
object.
Conclusion
In this blog post, we’ve seen examples of how to use the OK.Surf API with JavaScript to retrieve, filter, and add data. With these examples as a starting point, you should have a solid foundation to start building your application using the OK.Surf API.
Got any questions or feedback for us? Do let us know in the comments below!