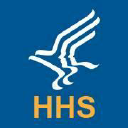
NPPES
HealthIntroducing NPI Registry API
NPI Registry is a free and public API service provided by the Centers for Medicare & Medicaid Services (CMS) that allows you to search for National Provider Identifier (NPI) information. This is useful for many purposes such as finding providers or validating the accuracy of medical information.
The API is provided in REST architecture, making it easy to integrate with your applications. In this blog post, we will explore how to make use of the NPI Registry API in JavaScript.
API Examples in JavaScript
Before you start using the NPI Registry API, you need to generate an API key which you will use to authenticate your requests. Once you have your API key, you can start exploring the different endpoints provided by the API.
Search by NPI
To search for a provider's information using their NPI, you can use the following JavaScript code:
const apiKey = 'YOUR_API_KEY_HERE';
const npi = '1234567890'; // replace with your desired NPI number
const apiUrl = `https://npiregistry.cms.hhs.gov/api/?version=2.1&number=${npi}&enumeration_type=NPI-1&api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
This will send a request to the NPI Registry API, using the provided API key and the NPI number you specified. The fetch
function returns a promise which is resolved with the API response as a JSON object.
Search by Name
You can also search for providers using their name. Here's an example:
const apiKey = 'YOUR_API_KEY_HERE';
const name = 'John Doe'; // replace with your desired name
const apiUrl = `https://npiregistry.cms.hhs.gov/api/?version=2.1&first_name=${name}&last_name=&city=&state=&taxonomy_description=&limit=1&api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
This code will return information about the provider with the name "John Doe". You can specify additional parameters such as city, state, and taxonomy description to filter the results further.
Search by Taxonomy Code
You can also search for providers using their taxonomy code. Here's an example:
const apiKey = 'YOUR_API_KEY_HERE';
const taxonomyCode = '207X00000X'; // replace with your desired taxonomy code
const apiUrl = `https://npiregistry.cms.hhs.gov/api/?version=2.1&taxonomy_description=${taxonomyCode}&limit=1&api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
This code will return information about providers with the specified taxonomy code.
Conclusion
In this blog post, we explored the NPI Registry API and how to use it in JavaScript. We covered three different ways of searching for provider information - by NPI, name, and taxonomy code.
The NPI Registry API is a powerful tool for anyone needing to access provider information, and it's easy to integrate with your own applications or systems. With the examples provided in this post, you should be well on your way to using this API in your own projects.