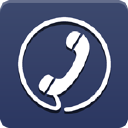
Numverify
Data ValidationUsing the Numverify API with JavaScript
The Numverify API is a public API that allows you to easily verify phone numbers and retrieve information about them. In this tutorial, we will explore how to use the Numverify API with JavaScript.
Getting started
First, you need to sign up for a free API key from Numverify. Once you have your API key, you can make requests to the Numverify API using JavaScript.
Making requests to the Numverify API
To make requests to the Numverify API, you can use the built-in fetch function in JavaScript. Here is an example code snippet that shows you how to make a request to the Numverify API and retrieve information about a phone number:
fetch('http://apilayer.net/api/validate', {
method: 'POST',
body: JSON.stringify({access_key: YOUR_API_KEY, number: PHONE_NUMBER}),
headers: {'Content-type': 'application/json'}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
In the above code, replace YOUR_API_KEY
with your actual API key, and PHONE_NUMBER
with the phone number you want to verify.
Handling the response
Once you make a request to the Numverify API, you will receive a response in JSON format. Here is an example response:
{
"valid": true,
"number": "14158586273",
"local_format": "4158586273",
"international_format": "+14158586273",
"country_prefix": "+1",
"country_code": "US",
"country_name": "United States of America",
"location": "San Francisco",
"carrier": "AT&T Mobility LLC",
"line_type": "mobile"
}
You can access the fields in the response just like you would with any JSON object in JavaScript. For example, you can access the country code like this:
console.log(data.country_code);
Conclusion
In this tutorial, we have explored how to use the Numverify API with JavaScript. By following these simple steps, you can easily verify phone numbers and retrieve information about them. If you have any questions or feedback, feel free to leave a comment below.