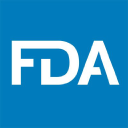
openFDA
HealthUsing the FDA Open API with JavaScript
The FDA Open API provides access to various datasets related to drug information, recalls, adverse events, and more. In this guide, we will explore how to use the FDA Open API with JavaScript, using example code snippets.
Setup
Before we can start using the FDA Open API with JavaScript, we need to obtain an API key. Follow these steps to obtain an API key:
- Go to the FDA Open API website and click on "Get API Key" in the top-right corner.
- Create an account or sign in if you already have one.
- Follow the steps to create an API key.
Once you have obtained an API key, you can start using the API.
Using the API
There are several API endpoints provided by the FDA Open API, each related to specific data sets. You can explore the available endpoints and their documentation on the API documentation page.
Retrieving drug information
To retrieve information about a specific drug, we can use the /drug/label.json
endpoint. Here is an example code snippet in JavaScript:
const api_key = 'YOUR_API_KEY';
const drug_name = 'aspirin';
fetch(`https://api.fda.gov/drug/label.json?search=${drug_name}&api_key=${api_key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code uses the fetch
function to make a GET request to the /drug/label.json
endpoint, with the search
parameter set to the value of drug_name
. We also include the api_key
parameter in the URL. The response is parsed as JSON and logged to the console.
Retrieving adverse events
To retrieve information about adverse events related to a specific drug, we can use the /drug/event.json
endpoint. Here is an example code snippet in JavaScript:
const api_key = 'YOUR_API_KEY';
const drug_name = 'aspirin';
fetch(`https://api.fda.gov/drug/event.json?search=${drug_name}&api_key=${api_key}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code is similar to the previous example, but with a different endpoint and different search parameters. The response will include data about adverse events related to the specified drug.
Conclusion
In this guide, we explored how to use the FDA Open API with JavaScript. We covered information retrieval for both drugs and adverse events. By following the examples presented in this guide, you should be able to start building applications that utilize the FDA Open API.