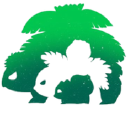
Pokémon TCG
Games & ComicsThe Pokémon TCG API allows developers to easily consume Pokémon card data in JSON format.
📚 Documentation & Examples
Everything you need to integrate with Pokémon TCG
🚀 Quick Start Examples
// Pokémon TCG API Example
const response = await fetch('https://pokemontcg.io', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the Pokemon TCG API in JavaScript
The Pokemon TCG API website provides a simple and easy way to access information about Pokemon trading card game cards, sets, and more. The API supports a variety of HTTP methods, including GET, POST, PUT, and DELETE, and returns results in JSON format.
Getting Started
First, you will need to register for an API access token. You can do this by visiting the Pokemon TCG API website and creating a new account.
Once you have an access token, you can begin making requests to the API using JavaScript. Here is an example of how to create a simple GET request in JavaScript:
fetch('https://api.pokemontcg.io/v2/cards', {
headers: {
'X-Api-Key': 'your_api_key_here'
}
})
.then(response => response.json())
.then(data => console.log(data));
This code uses the fetch
function to make a GET request to the https://api.pokemontcg.io/v2/cards
endpoint. The headers
object includes the API access token that you obtained earlier.
The then
methods are used to handle the response data. The first then
method converts the response to JSON format, and the second then
method logs the data to the console.
Example Requests
Here are some additional examples of requests you can make to the Pokemon TCG API using JavaScript:
Get a Specific Card
fetch('https://api.pokemontcg.io/v2/cards/xy7-54', {
headers: {
'X-Api-Key': 'your_api_key_here'
}
})
.then(response => response.json())
.then(data => console.log(data));
This code retrieves information about the card with ID xy7-54
. Replace your_api_key_here
with your actual API access token to make the request.
Search for Cards
fetch('https://api.pokemontcg.io/v2/cards?q=name:charizard', {
headers: {
'X-Api-Key': 'your_api_key_here'
}
})
.then(response => response.json())
.then(data => console.log(data));
This code searches for all cards with the name "Charizard". Replace your_api_key_here
with your actual API access token to make the request.
Get a List of Sets
fetch('https://api.pokemontcg.io/v2/sets', {
headers: {
'X-Api-Key': 'your_api_key_here'
}
})
.then(response => response.json())
.then(data => console.log(data));
This code retrieves a list of all the sets available in the Pokemon TCG. Replace your_api_key_here
with your actual API access token to make the request.
Conclusion
Using the Pokemon TCG API in JavaScript is simple and easy. With access to a wide range of information about Pokemon trading card game cards, sets, and more, you can create powerful applications that can help you and your users get the most out of the game.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes