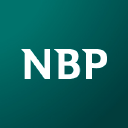
Poland
Currency Exchange'--- title: "Working with the NBP API in JavaScript" date: 2022-05-16
The National Bank of Poland (NBP) provides a public API for accessing data on the Polish currency exchange rates, gold, and other banking information. In this blog post, we will explore how to use the NBP API in JavaScript and provide some code examples.
Getting Started
First, we need to get an API key from the NBP. You can do this by visiting the NBP API website and filling out the registration form. Once you have your API key, you can begin making requests.
Exchange Rates
One of the most popular uses of the NBP API is to retrieve exchange rates. To do this, we need to make a GET
request to the API endpoint for exchange rates. Here's an example of how to do this in JavaScript:
const api = 'https://api.nbp.pl/api/exchangerates/rates/A/usd/';
const apiKey = '{YOUR_API_KEY}';
const url = api + '?format=json' + '&apiKey=' + apiKey;
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are retrieving the exchange rate for US dollars. We're using the fetch()
method to make the request and the then()
method to handle the response. Note that we've also included our API key in the request.
Gold Prices
Another useful aspect of the NBP API is its ability to provide gold prices. To retrieve gold prices, we need to make a GET
request to the API endpoint for gold prices. Here's an example:
const api = 'https://api.nbp.pl/api/cenyzlota/';
const apiKey = '{YOUR_API_KEY}';
const url = api + '?format=json' + '&apiKey=' + apiKey;
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're retrieving the current price of gold. Once again, our API key is included in the request.
Conclusion
In this blog post, we've explored how to use the NBP API in JavaScript to retrieve exchange rates and gold prices. The NBP API provides a wealth of data, and with a little bit of code, we can easily access it to build useful applications. If you're interested in learning more about the NBP API, be sure to check out their documentation.