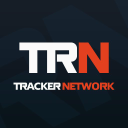
PUBG Tracker API
Games & ComicsShort Introduction to the PUBG Tracker API
If you're a big fan of the popular battle royale game, PlayerUnknown's Battlegrounds (PUBG), then the PUBG Tracker API documentation is definitely worth checking out. With the PUBG Tracker API, you can access a lot of interesting data about the game, including player stats, matches, seasons, leaderboards, and more.
To start using the PUBG Tracker API, you'll first need to create an API key. You can do this by registering for a free account on the PUBG Tracker website. Once you have your API key, you can perform various HTTP requests to retrieve data from the API.
ExampleCode in JavaScript
Here are a few examples of how you can use the PUBG Tracker API in JavaScript, using the popular Axios library to handle HTTP requests:
Retrieving a Player's Stats
const axios = require('axios');
const API_KEY = 'your-api-key-here';
const getPlayerStats = async (playerName) => {
const response = await axios.get(`https://pubgtracker.com/api/profile/pc/${playerName}`, {
headers: {
'TRN-Api-Key': API_KEY
}
});
const { data: { stats } } = response;
return stats;
};
// Example usage: retrieve stats for player "shroud"
getPlayerStats('shroud')
.then((stats) => console.log(stats))
.catch((err) => console.error(err));
Retrieving Match Data
const axios = require('axios');
const API_KEY = 'your-api-key-here';
const getMatchData = async (matchId) => {
const response = await axios.get(`https://pubgtracker.com/api/matches/pc/${matchId}`, {
headers: {
'TRN-Api-Key': API_KEY
}
});
const { data } = response;
return data;
};
// Example usage: retrieve data for match "542b07b5-6c06-47bc-84c7-6cf08b6b2f96"
getMatchData('542b07b5-6c06-47bc-84c7-6cf08b6b2f96')
.then((matchData) => console.log(matchData))
.catch((err) => console.error(err));
Retrieving Leaderboard Data
const axios = require('axios');
const API_KEY = 'your-api-key-here';
const getLeaderboardData = async (region, gameMode, count) => {
const response = await axios.get(`https://pubgtracker.com/api/leaderboards/${region}/${gameMode}?limit=${count}`, {
headers: {
'TRN-Api-Key': API_KEY
}
});
const { data: { rows } } = response;
return rows;
};
// Example usage: retrieve top 10 players in duo mode for NA region
getMatchData('na', 'duo', 10)
.then((leaderboard) => console.log(leaderboard))
.catch((err) => console.error(err));
Conclusion
Using the PUBG Tracker API, you can easily access a wealth of data about PUBG players and matches. With these JavaScript examples, you can start experimenting with the API and leveraging its data to build useful applications or tools.