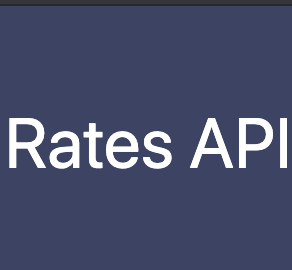
📚 Documentation & Examples
Everything you need to integrate with ratesapi
🚀 Quick Start Examples
// ratesapi API Example
const response = await fetch('https://ratesapi.io', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using RatesAPI.io Public API in JavaScript
If you're looking for a reliable, easy-to-use public API for currency exchange rates, RatesAPI.io might be just what you need! RatesAPI.io provides up-to-date exchange rate data for over 30 currencies.
Here's how you can use the RatesAPI.io public API in your JavaScript projects.
Getting Started
Before you can start using the RatesAPI.io public API, you'll need to sign up for a free API key. Visit the RatesAPI.io website to sign up.
Once you have your API key, you're ready to start making requests!
Making Requests
You can get the latest exchange rates for a specific currency by making a GET request to the RatesAPI.io API with the following URL:
https://api.ratesapi.io/api/latest?base=USD&symbols=EUR
In this example, the base
parameter is set to USD
to get exchange rates relative to the US Dollar, and the symbols
parameter is set to EUR
to get the exchange rate for the Euro.
Here's an example of how you can make this request in JavaScript:
const apiUrl = "https://api.ratesapi.io/api/latest?base=USD&symbols=EUR";
fetch(apiUrl, { headers: { "Content-Type": "application/json" } })
.then(response => response.json())
.then(data => console.log(data));
This code uses the fetch
method to make the GET request to the API. The headers
parameter sets the content type to JSON, and the response is converted to JSON format with the json
method.
If the request is successful, the data returned by the API will be logged to the console.
Using Historical Rates
If you need historical exchange rate data for a specific date, you can make a GET request to the API with the following URL:
https://api.ratesapi.io/api/2021-09-01?base=USD&symbols=EUR
In this example, the date is set to September 1, 2021. You can replace this date with any date in the format YYYY-MM-DD
.
Here's an example of how you can make a request for historical exchange rates in JavaScript:
const apiUrl = "https://api.ratesapi.io/api/2021-09-01?base=USD&symbols=EUR";
fetch(apiUrl, { headers: { "Content-Type": "application/json" } })
.then(response => response.json())
.then(data => console.log(data));
If the request is successful, the data returned by the API for the specified date will be logged to the console.
Conclusion
Using the RatesAPI.io public API is a simple and effective way to get up-to-date exchange rate data for your JavaScript projects. With just a few lines of code, you can make requests and retrieve data for a wide range of currencies.
Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes