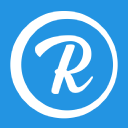
Rebrandly
URL ShortenersPublic API Docs for Rebrandly API: Getting Started with JavaScript
Rebrandly API is an easy-to-use, cloud-based API that enables developers to programmatically create short links for their applications. With Rebrandly API, it is effortless to build branded links, track clicks, and manage links in real-time.
In this blog, we'll cover the basics of using Rebrandly API with JavaScript. We'll provide some sample code and cover the most commonly used endpoints of this API, including shortening a link, retrieving links' information, and setting up custom domains to make the link more branded.
Authentication
Before we start using the Rebrandly API, we need to authenticate ourselves with the server using the API key. To generate your API key, sign up for an account on the Rebrandly website and navigate to the "API Keys" tab under the "Profile" menu.
Once you have your API key, add the following code to your JavaScript file:
const apiKey = "Your_API_Key";
const headers = { Accept: "application/json", "Content-Type": "application/json", apikey: apiKey }
Shortening a Link
Creating a short link is the main use case of the Rebrandly API. Here's an example of how to create a short link:
const requestOptions = {
method: "POST",
headers: headers,
body: JSON.stringify({
destination: "https://www.rebrandly.com/",
domain: { fullName: "rebrand.ly" }
})
};
fetch("https://api.rebrandly.com/v1/links", requestOptions)
.then(response => response.json())
.then(result => console.log(result.shortUrl))
.catch(error => console.log('error', error));
In the above code, we are making a POST request to the Rebrandly API endpoint "https://api.rebrandly.com/v1/links" to create a short URL.
Retrieving Links' Information
The Rebrandly API also allows you to retrieve links' information. Here's an example of how to retrieve a single link's information using the link ID:
const linkId = "Your_Link_Id";
const requestOptions = {
method: 'GET',
headers: headers,
};
fetch(`https://api.rebrandly.com/v1/links/${linkId}`, requestOptions)
.then(response => response.json())
.then(result => console.log(result.destination))
.catch(error => console.log('error', error));
Setting up a Custom Domain
Rebrandly API allows developers to configure their custom domain so that their shortened URL appears branded. Here's an example of how to set up a custom domain:
const requestOptions = {
method: "POST",
headers: headers,
body: JSON.stringify({
fullName: "Your_Custom_Domain_Name"
})
};
fetch("https://api.rebrandly.com/v1/account/domains", requestOptions)
.then(response => response.json())
.then(result => console.log(result.fullName))
.catch(error => console.log('error', error));
In the above code, we are making a POST request to the "https://api.rebrandly.com/v1/account/domains" endpoint to add a custom domain.
Conclusion
In this blog, we have covered how to use Rebrandly API with JavaScript. Whether you want to create short URLs, retrieve link information or set up custom domains, Rebrandly API makes it straightforward and accessible for developers. We hope this blog has been informative and helps you get started with Rebrandly API.