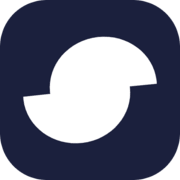
ShrtCode
URL ShortenersIntro to shrtco.de API
shrtco.de is a free to use URL shortening API that allows developers to shorten long URLs to a much shorter and manageable format. With a simple HTTP GET request, you can shorten any long URL using the shrtco.de API.
This document is an overview of the shrtco.de API and how to use it in JavaScript.
Getting started with shrtco.de API
To get started with the shrtco.de API, you will need an API key. It's very simple to get one - just visit the shrtco.de website and register for a free account.
Once you have an API key, you can start making HTTP GET requests to the shrtco.de API. The API will return a shortened URL in JSON format.
Here are some examples of shrtco.de API calls in JavaScript:
Example 1
async function shortenUrl(longUrl, apiKey) {
const res = await fetch(
`https://api.shrtco.de/v2/shorten?url=${longUrl}`,
{
headers: {
'Content-Type': 'application/json',
'apikey': apiKey,
},
}
);
if (!res.ok) {
const {status, statusText} = res;
throw new Error(`Error: ${status} ${statusText}`);
}
const data = await res.json();
return data.result.short_link;
}
This function takes in a long URL and an API key and returns a shortened URL. Here's how you can use this function:
const apiKey = 'YOUR_API_KEY';
const longUrl = 'https://www.google.com/';
const shortUrl = await shortenUrl(longUrl, apiKey);
console.log(shortUrl); // 'https://shrtco.de/abc123'
Example 2
async function shortenUrls(longUrls, apiKey) {
const urls = longUrls.map((url) => `url=${encodeURIComponent(url)}`);
const query = urls.join('&');
const res = await fetch(
`https://api.shrtco.de/v2/shorten?${query}`,
{
headers: {
'Content-Type': 'application/json',
'apikey': apiKey,
},
}
);
if (!res.ok) {
const {status, statusText} = res;
throw new Error(`Error: ${status} ${statusText}`);
}
const data = await res.json();
return data.result.map(({original_link, short_link}) => ({
original_link,
short_link,
}));
}
This function takes in an array of long URLs and an API key and returns an array of objects that contain the original URL and the shortened URL.
const apiKey = 'YOUR_API_KEY';
const longUrls = [
'https://www.google.com/',
'https://www.amazon.com/',
'https://www.github.com/',
];
const shortUrls = await shortenUrls(longUrls, apiKey);
console.log(shortUrls);
/*
[
{original_link: 'https://www.google.com/', short_link: 'https://shrtco.de/abc123'},
{original_link: 'https://www.amazon.com/', short_link: 'https://shrtco.de/def456'},
{original_link: 'https://www.github.com/', short_link: 'https://shrtco.de/ghi789'},
]
*/
Conclusion
In this blog post, we've covered how to use the shrtco.de API to generate shortened URLs using JavaScript. The API is simple to use, and you can get started with it in just a few minutes. If you're building an application that requires URL shortening, give the shrtco.de API a try!